Python List Manipulation: Convert Lists to Strings
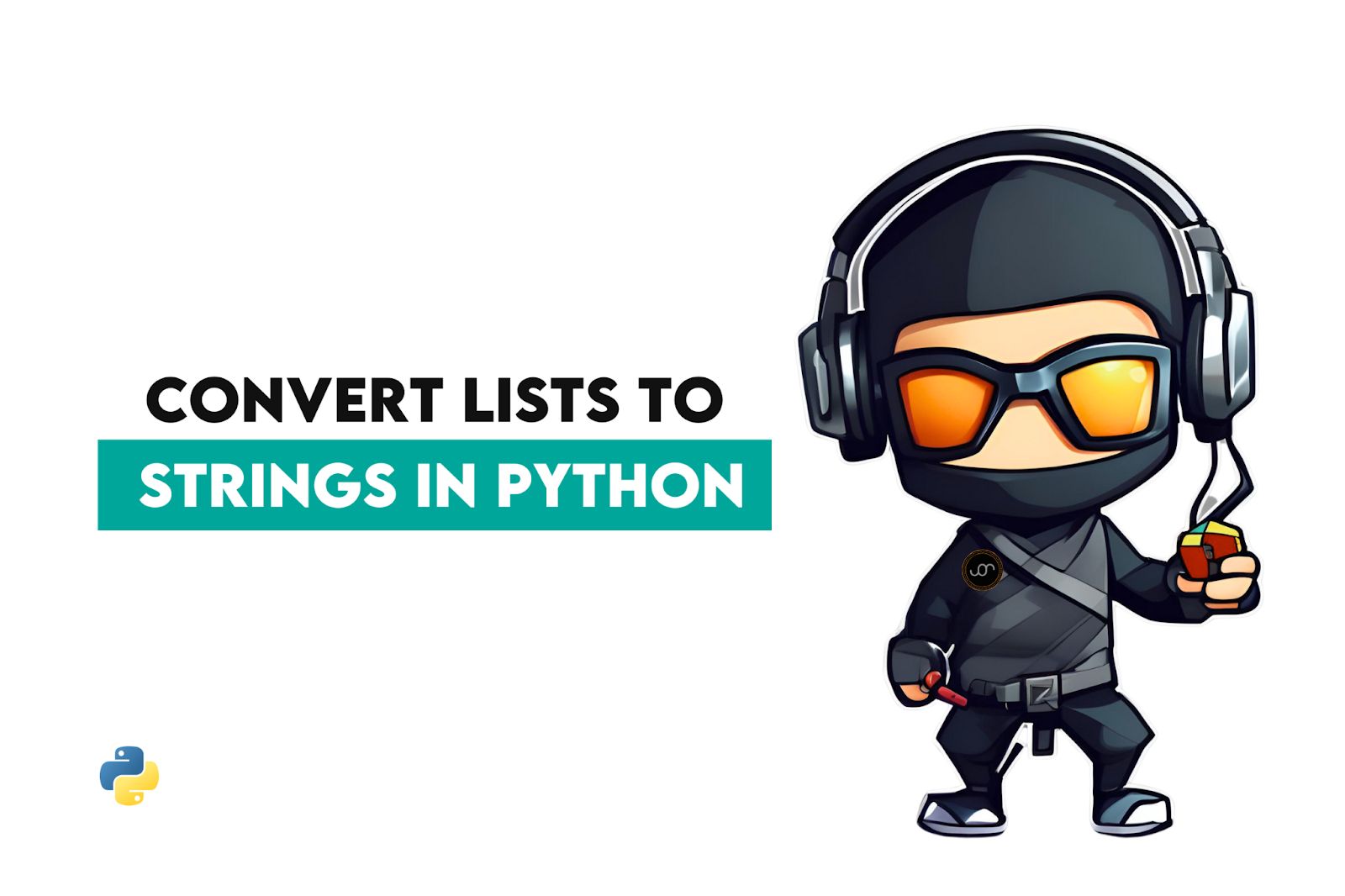
- Written by:
Nathan Rosidi
Convert list to string Python using practical, real-world techniques. Learn clean methods, handle edge cases, and apply them in logging, files, and UIs.
Ever stared at a jumbled list in Python and thought, “How do I turn this mess into one neat string?”
Lists often hold valuable data, but they’re not always in the format you need—especially when working with files, APIs, or user interfaces.
I’ll show you multiple ways to convert lists to strings in python, highlight common pitfalls, and share real-world use cases where this comes in handy.
Why Convert Lists to Strings in Python?
Python lists are incredibly flexible, but they’re not accepted as input everywhere.
Sometimes, you need to present data in a human-readable format—like displaying tags in a UI or saving items to a CSV file.
You might also run into APIs or web forms that only accept strings, not arrays. Even writing to logs or debug outputs becomes cleaner when you format lists as strings.
So whether you’re building a user interface, exporting data, or just tidying up console output, this conversion step becomes surprisingly essential. It’s a tiny transformation that unlocks big compatibility and clarity across your codebase, like all other Python list methods, including Python add to list.
Methods to Convert Lists to Strings in Python
There are several ways to turn a list into a string, and your choice often depends on what's in the list and how you want the result to look.
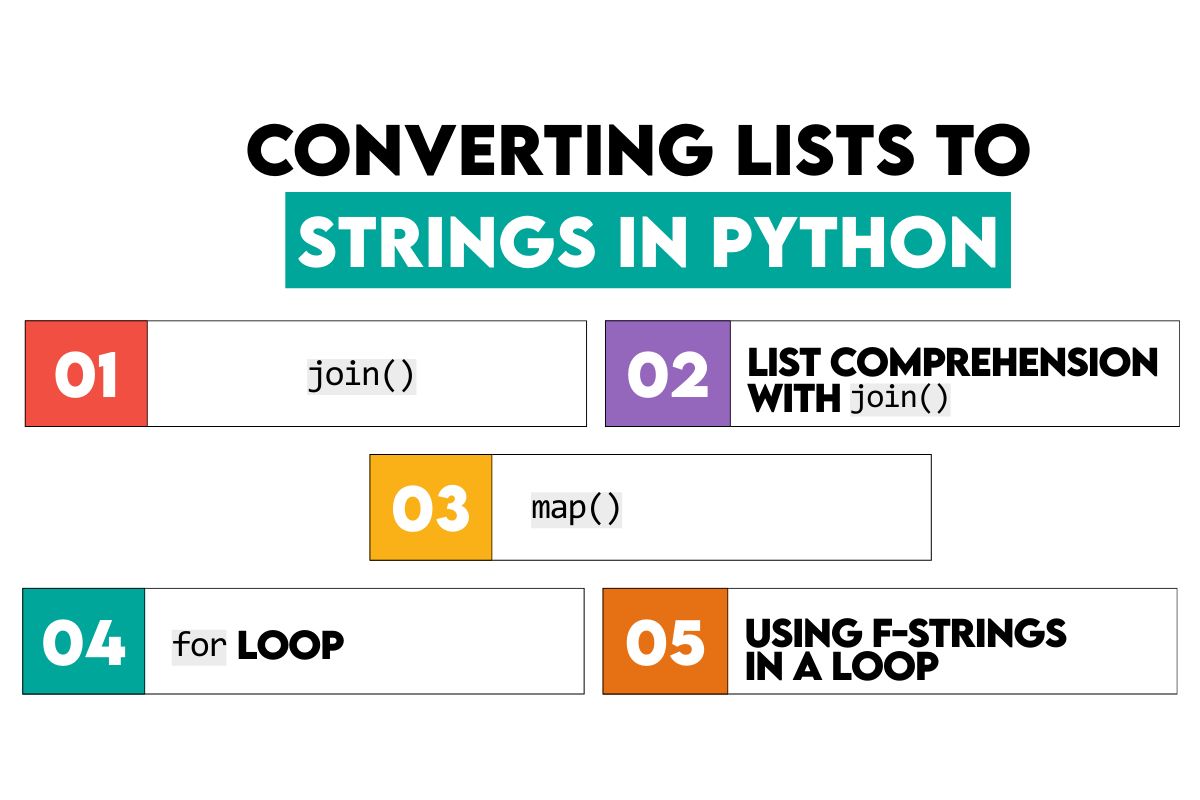
Let’s break down each method, walk through the logic behind it, and see how it performs in action.
1. Using join() – The Go-To Method
This is the most common and efficient method when your list contains only string elements.
It uses a separator—like a comma, space, or dash—to combine items into a single string.
In the example, we specify the separator (in our case, a comma and a blank space) that will separate the items and join them using the .join()
method.
my_list = ['Karl', 'Friedrich', 'Rosa', 'Angela']
result = ', '.join(my_list)
print(result)
Here’s the output.

2. Using List Comprehension With join() – When You’ve Got Mixed Types
If your list includes integers, floats
, or None
, join()
will raise an error unless everything is a string. By wrapping each item in str()
– a built-in Python function for representing and creating strings – using comprehension, you avoid errors and keep things flexible.
Below is an example of how to do that.
mixed_list = ['Age:', 30, 'Height:', 170]
result = ' '.join(str(item) for item in mixed_list)
print(result)
Here’s the output.

3. Using map() – A Functional Twist
For a clean and functional approach, map()
is a powerful option. It converts each element into a string and then hands the result to join()
without needing a list comprehension.
Here’s an example. We use a dash as a separator. In the join()
function, we embed map()
. We specify two arguments. The first one is the function we want to apply to every item in the list or any other iterable. In our case, this is str.
The second argument in map() is an iterable that we want to transform.
items = [3, 4, 2, 1]
result = '-'.join(map(str, items))
print(result)
The code outputs this.

4. Using a For Loop – When You Want Full Control
When you want more flexibility—like handling formatting or adding custom logic – a loop gives you that edge. You build the string manually and can tweak it any way you like.
Here’s an example. In the code, result = ''
creates an empty string where the final output will be built. In the for color in colors:
part, we’re looping through each item in the list one by one.
With each loop, we add the color plus a separator (' | ')
to the result string by result += color + ' | '
.
colors = ['red', 'green', 'blue']
result = ''
for color in colors:
result += color + ' | '
print(result)
Here’s the output.

Notice the extra ' | '
at the end? We’ll address that in one of the following sections. For now, let’s leave the output as is.
5. Using F-Strings in a Loop – For Structured Formatting
If you're working with HTML, XML, or some custom layout, f-strings allow you to style each item as you go. You can insert tags, wrappers, or even conditionals as you build the string.
Here’s an example. The code is similar to the previous one. But, here’s the magic line: result += f'<p>{name}</p>\n';
it wraps each name in an HTML <p> tag (for paragraph). The f'<p>{name}</p>'
part is an f-string, which means it inserts the value of name inside the tag, then \n
adds a newline character to make the output readable line-by-line.
names = ['Karl', 'Friedrich', 'Rosa', 'Angela']
result = ''
for name in names:
result += f'<p>{name}</p>\n'
print(result)
Here’s the output.
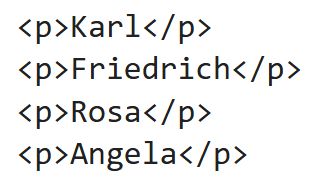
Common Challenges and How to Handle Them
List-to-string conversion sounds easy – until it isn’t.
Things get tricky when your list isn’t made up of perfect strings. From None values to special characters and unexpected data types, Python will raise errors or give weird output unless you handle these issues properly.
Let’s explore some common headaches and how to fix them.

1. Mixed Data Types – When Lists Aren’t Just Strings
The join in Python only works when every element in the list is a string. If your list contains numbers, booleans, or especially None, Python won’t know what to do—and it’ll raise a TypeError
.
Even if you convert everything using str()
, the output may still contain things you don’t want—like "None"
showing up in your final string. That’s why the real challenge isn’t just conversion, but also filtering out unwanted data.
The best solution? Use a generator expression with a conditional check. It converts each item to a string but only if it’s not None. This keeps your final string clean and intentional.
data = ['Score:', 98, None, 'Passed:', True]
result = ' '.join(str(item) for item in data if item is not None)
print(result)
Here’s the output.

2. Extra Spaces and Trailing Characters
Sometimes, when building a string manually – especially inside loops – you end up with an unwanted character at the end (like a trailing comma or pipe). This might not crash your code, but it definitely makes the output look sloppy.
To fix this, you can remove the trailing characters using .rstrip().
In the for
loop example, we ended up with the trailing separator – specifically the last ' | '
at the end of the string in the output. We’ll now fix this with .rstrip()
. This method trims characters from the end of the string.
colors = ['red', 'green', 'blue']
result = ''
for color in colors:
result += color + ' | '
result = result.rstrip(' | ')
print(result)
Here’s the output.

3. Lists Containing Special Characters
Special characters – like newlines (\n
), tabs (\t
), or quotation marks – can cause formatting issues or confusion. For example, newlines may break your layout, and quotes might be misinterpreted in HTML or logs.
To handle this, use repr()
to represent each item exactly as Python sees it. It escapes characters and makes things easier to debug or store.
In the code below, a list is filled with special characters: newline, tab, and quotes.
The repr(item) for item in specials
part turns each item into its string representation, showing escaped characters like \n
and \t
.
The escaped strings are then joined by join()
using a pipe separator (|
) for visibility.
specials = ['hello\n', 'world\t', '"quoted"']
result = ' | '.join(repr(item) for item in specials)
print(result)
Here’s the output.

This makes it super clear what’s actually inside your string, which is helpful for debugging or creating safe output for logs and files.
Practical Applications of Converting Lists to Strings in Python
List-to-string conversion isn’t just a coding trick – it’s something you’ll use constantly in real-life projects. Whether you’re exporting data, improving logs, formatting user interfaces, or working with APIs, the ability to flatten and convert lists to strings in python helps make your data readable, transferable, and functional.
Below are several practical scenarios where this conversion becomes essential.
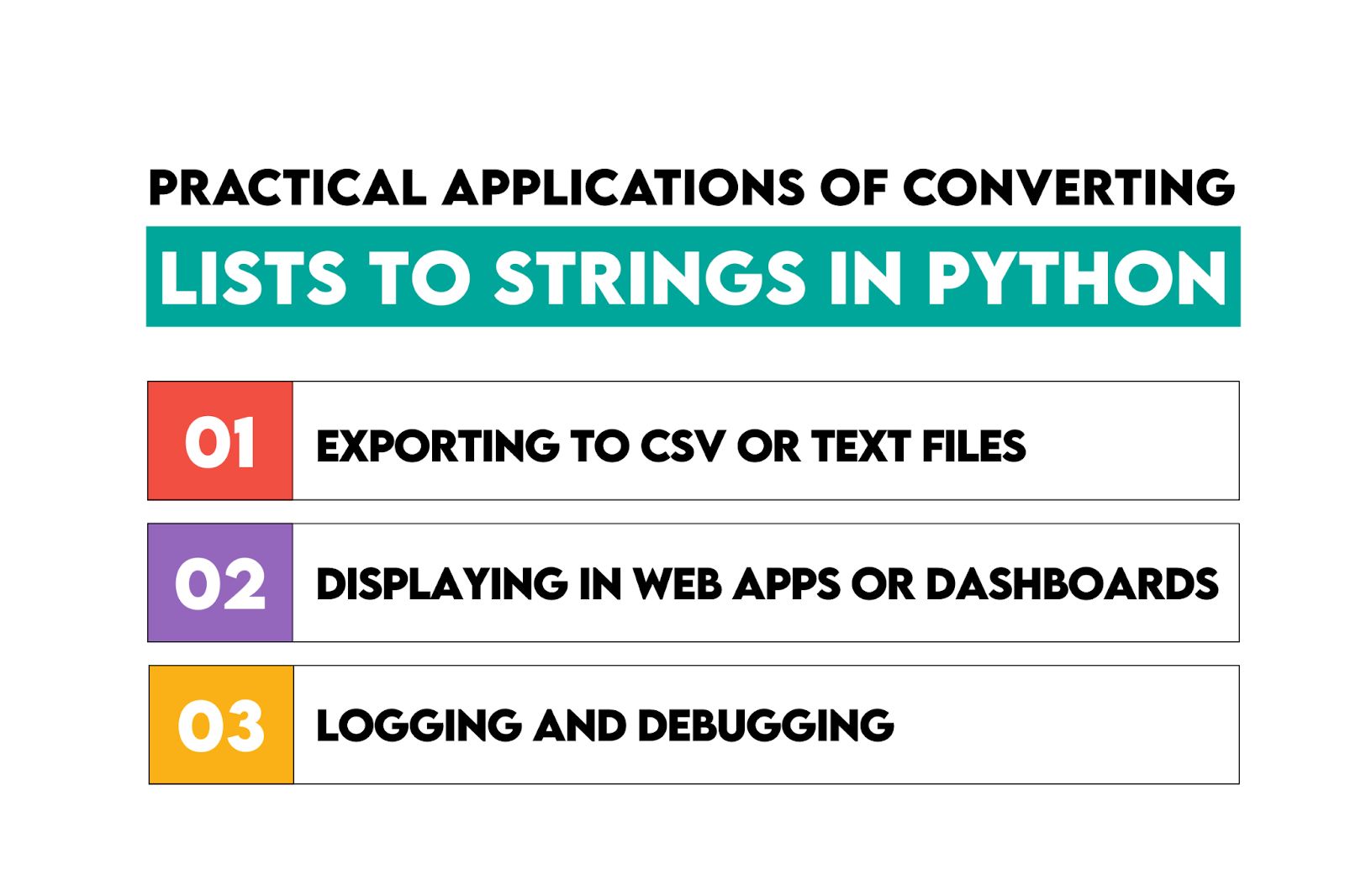
1. Exporting to CSV or Text Files
When you're writing data to a .csv file or a .txt document, each row often needs to be a single line of text.
Lists work well for storing row data temporarily, but before saving them, you need to flatten and convert that list into a string. This method keeps your files clean, properly delimited, and readable by Excel or other tools.
Here’s an example. The code creates a list named row
. The line with open('data.csv', 'w') as f:
opens a new file named data.csv
in write mode ('w'
).
Because integers can’t be joined as-is, we convert each item in the list to a string with this: str(item) for item in row
. Then, we join all the string items using join()
and a comma separator to create a single line. The write()
function writes that single line into the file data.csv
.
row = ['ID123', 'Alice', 25]
with open('data.csv', 'w') as f:
f.write(','.join(str(item) for item in row))
To read the file back, add this code:
with open('data.csv', 'r') as f:
print(f.read())
Here’s what the output looks like.

2. Displaying in Web Apps or Dashboards
In user interfaces, like dashboards or admin panels, you may need to show multiple roles, tags, or labels as a single string. Converting a list of user roles into a single display string makes the UI more compact and easier to read. This is perfect for admin panels, profile views, or badge systems.
Here’s the code example.
The join()
method takes each item in the list and joins them into one string, separated by commas and a space.
The print(f"User roles: {display}")
line uses an f-string to embed the display string into a larger message.
roles = ['admin', 'editor', 'viewer']
display = ', '.join(roles)
print(f"User roles: {display}")
The code prints this output. It’s clean and perfectly formatted for showing in a UI, log, or console.

Conclusion
Converting lists to strings in Python might seem small, but it opens up a world of clean output, better formatting, and flexible data handling.
You’ve now seen multiple ways to do it, plus how to handle tricky edge cases and apply the techniques in real scenarios.
The right method depends on what your list looks like and where that string is going. Whether it’s logging, saving, or showing – there’s a tool here that’ll get the job done fast and clean.
FAQs
1. What happens if my list contains None?
Python’s join()
method can’t handle None directly – it expects all items to be strings. If you pass a list with None, it’ll throw a TypeError
.
The solution is to use a comprehension or map()
to convert all items to strings.
2. How do I handle lists with special characters?
Special characters like newlines (\n
), tabs (\t
), or quotes ("
) can mess with formatting. If you're outputting to a file or console, use repr()
or escape them manually.
3. Which method is the fastest to convert a list to a string in Python?
For pure string lists, ''.join()
is hands-down the fastest – it’s built into Python and highly optimized.
If you’re dealing with mixed types, map(str, list)
inside join()
is both fast and clean.
Share