Looping Through Lists in Python: A Comprehensive Tutorial
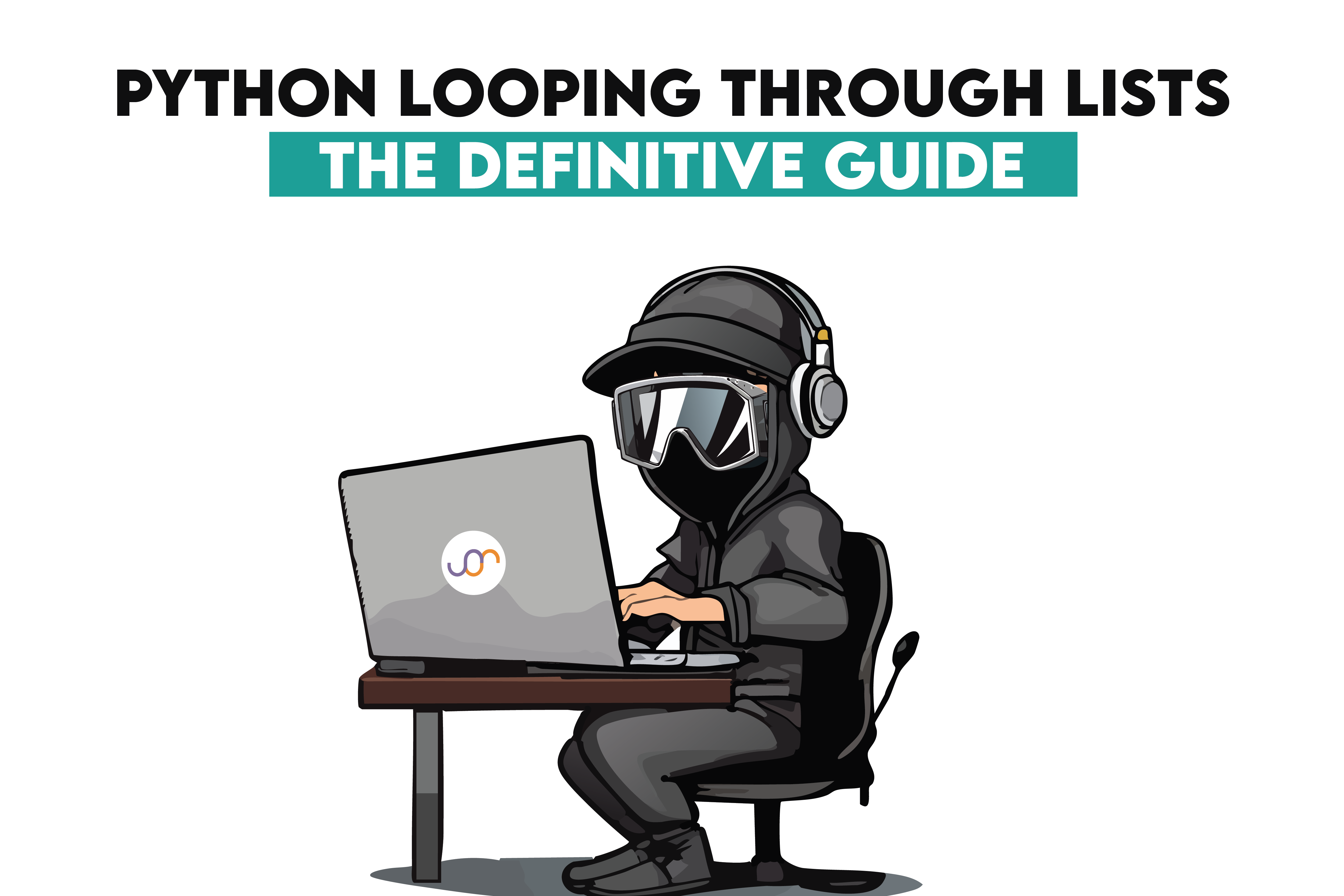
Categories:
- Written by:
Nathan Rosidi
Learn how to loop through a list in Python using real Uber data, practical techniques, and performance-friendly tips.
If you can loop wisely, Python lists aren ’t just simple containers but portals for tackling meaningful data problems.
In this article, I will try to teach you some list-looping techniques through a real Uber data challenge. You will learn how mastering lists can help you with bonus payouts and avoid missing out on trips.
What Are Lists in Python?
You can think of a Python list like a bag of assorted snacks—you can indulge yourself with what you find there, one at a time, or you can snack on the whole bag at once.
Also, a list is simply a collection of items enclosed in [], like this. These could be numbers, strings, dictionaries, or even other lists.
You can change them, add a couple of extras, or remove some. Data structures like Python lists offer flexibility and ease of use—precisely what both novices and professionals love about them.
They preserve their order, so as you traverse them, it feels like you’re traversing through the data in the exact order that was added.
If you want to discover more, check out our post on Python lists.
Why Loop Through a List?
You have a list of hundreds of Uber drivers, all with trip counts, ratings, and hours logged. You wouldn't want to do all of that manually, right?
Looping lets you get into that list like a boss — checking conditions, screening drivers for born at midnight time, or just looking to see who busted through twelve trips on a Saturday.
It's not only effective but solid. It’s how you turn disorganized data into meaningful decisions.
Common Ways to Loop Through Lists in Python
Python offers an infinite number of looping options for lists. Some are fast, some graceful, and some might just leave you to go, “Whoa.”
For this one, we are going to Allegro, a real-life data project.
Case Study: Looping Through Lists with Real Uber Driver Data
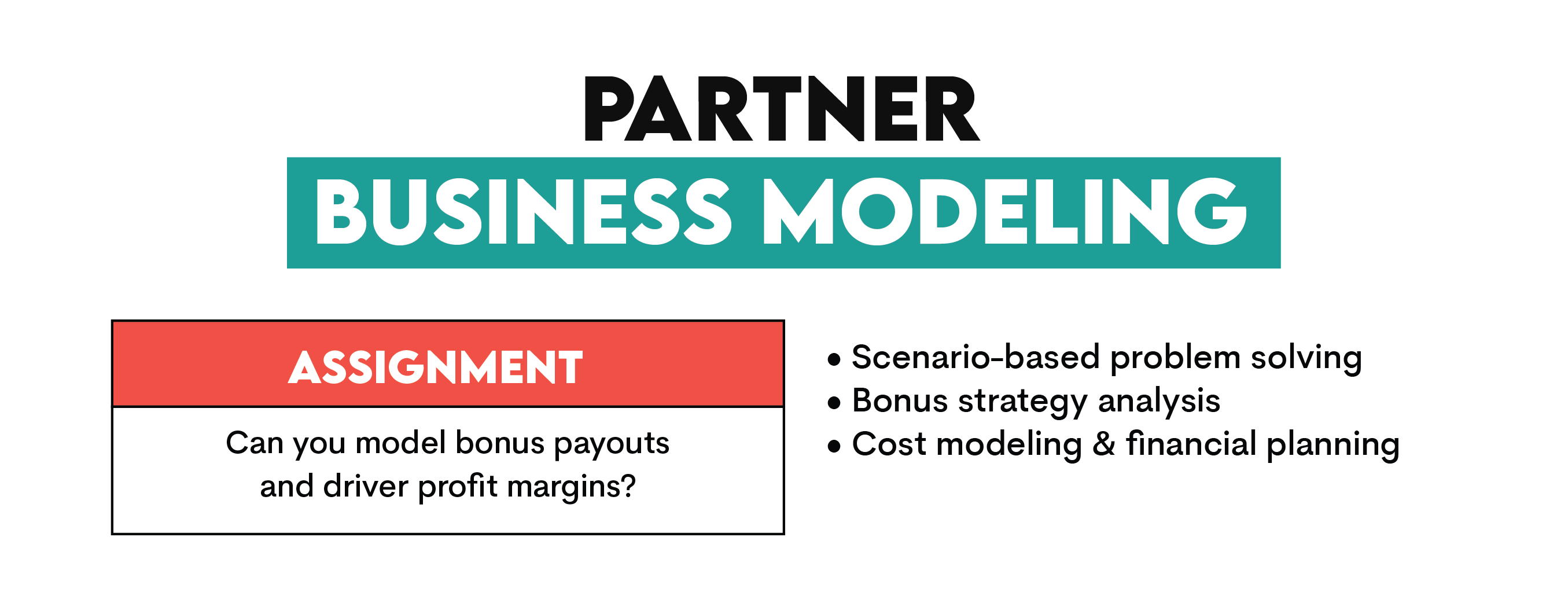
Link to the project: https://platform.stratascratch.com/data-projects/partner-business-modeling
Each row tells a story: how many rides a driver completed, how long they were on duty, how many requests they accepted, and what riders thought of them—will guide us. Let's go through this together.
Using for Loop
You print each driver's name if they have completed 20 or more trips. This is very simple yet very frequent. You walk step by step through the list—clean, simple, and completely beginner-friendly—the `for` loop.
Here is the code.
Import pandas as pd
df = pd.read_csv('dataset_2.csv')
drivers = df.to_dict('records')
for driver in drivers:
if driver['Trips Completed'] >= 20:
print(f"{driver['Name']} completed {driver['Trips Completed']} trips")
Here is the output.
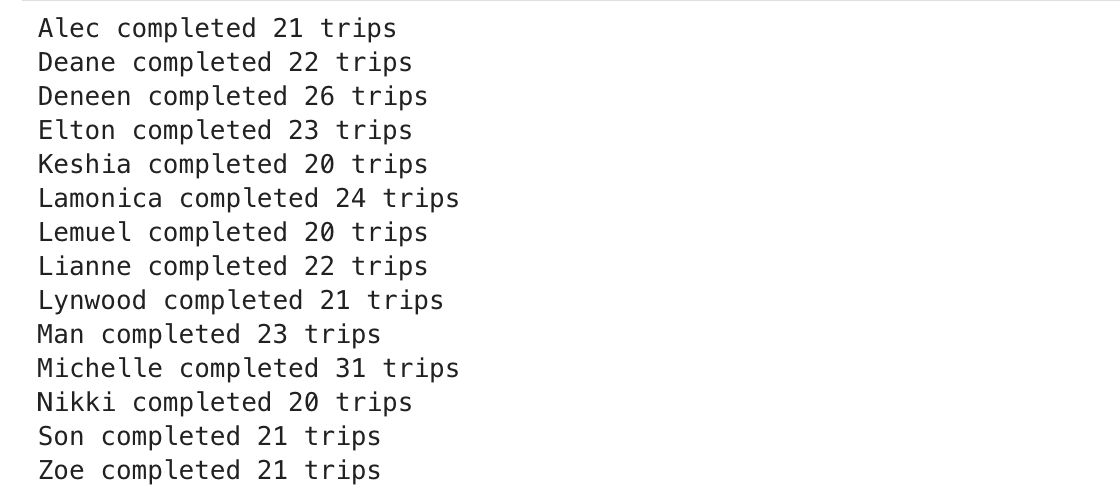
This will print a list of drivers who crossed that 20-trip threshold—precisely what Option 2 in the Uber bonus scenario cares about.
Using while Loop
You don’t use `while` loops as often in data tasks—but when you do, it's usually for more dynamic conditions. Say you want to keep looping until you find five drivers with a rating over 4.9.
Here is the code.
top_rated = []
i = 0
while len(top_rated) < 5 and i < len(drivers):
if drivers[i]['Rating'] > 4.9:
top_rated.append(drivers[i]['Name'])
i += 1
print("Top-rated drivers:", top_rated)
Here is the output.

The loop keeps going until it finds five top-tier drivers—or runs out of drivers altogether. This lets you stop early instead of checking every single row.
Using List Comprehensions
List comprehensions are snappy. If you want a clean, one-liner to grab names of drivers online for more than 8 hours, this is your move. Here is the code.
long_haul_names = [d['Name'] for d in drivers if d['Supply Hours'] >= 8]
print(long_haul_names)
Here is the output.

Just the names. No fluff. These folks stayed on the road all day long.
Using the enumerate() Function
Let’s say you want not just the driver but which row they appeared on. Maybe for logging or flagging. `enumerate()` adds an index while you loop. Here is the code.
for idx, driver in enumerate(drivers):
if driver['Rating'] < 4.5:
print(f"Row {idx}: {driver['Name']} has a low rating of {driver['Rating']}")
Here is the output.

Because sometimes context matters. Seeing where in the dataset an issue appears can help track patterns—or bad records.
Using zip() for Multiple Lists
Let’s say you split your data. You’ve got a list of names and another with trip counts. You want them together, side by side.
Here is the code.
names = df['Name'].tolist()
trips = df['Trips Completed'].tolist()
for name, trip_count in zip(names, trips):
print(f"{name} completed {trip_count} trips")
Here is the output’s first part. (It continues for each driver.)
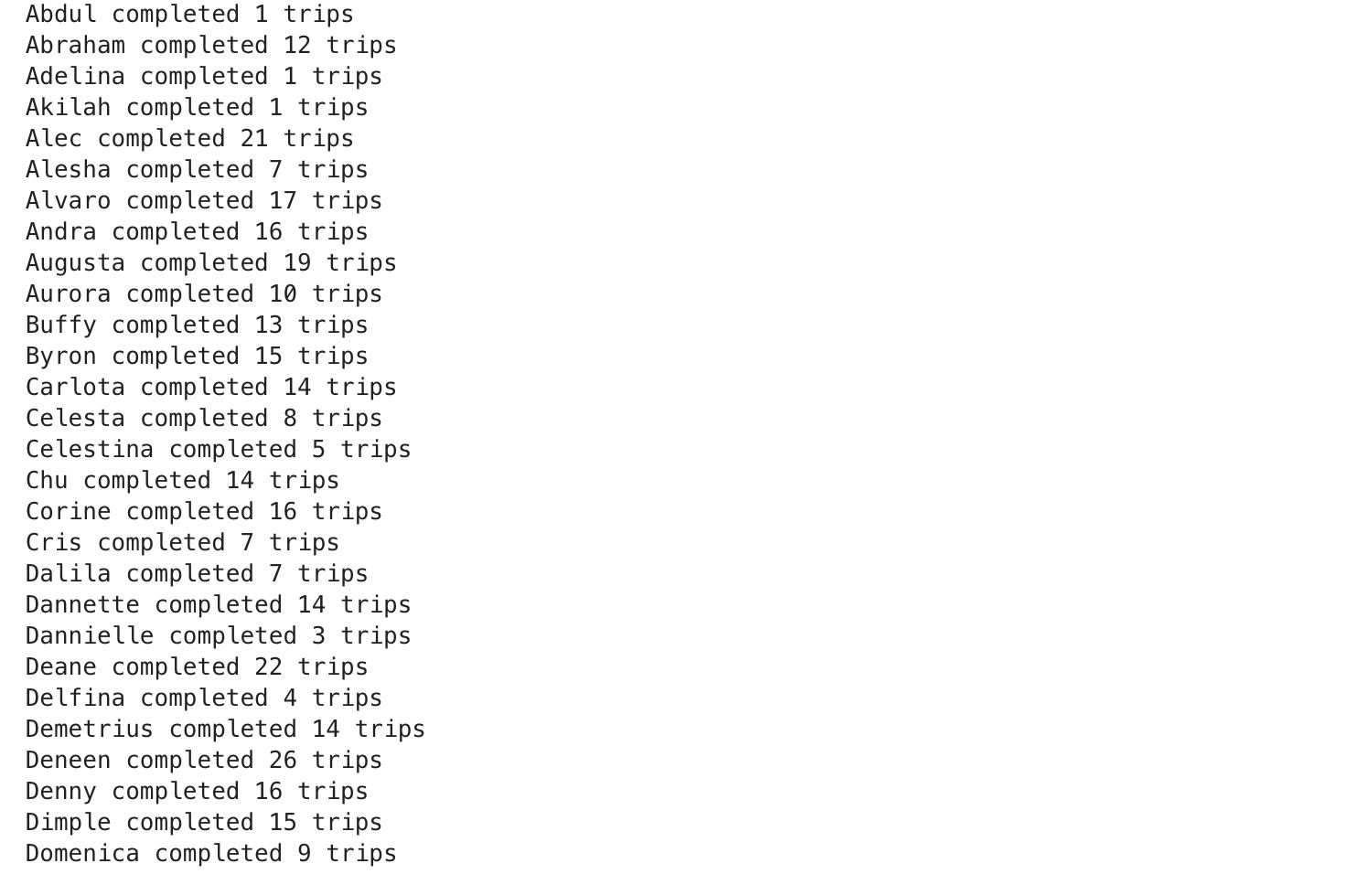
Pairs of names with how many trips they logged. This comes in handy when you’re building side-by-side comparisons.
Advanced Techniques for Looping Through Lists
Let’s look at advanced techniques like finding elements with loops or using nested loops and generators.
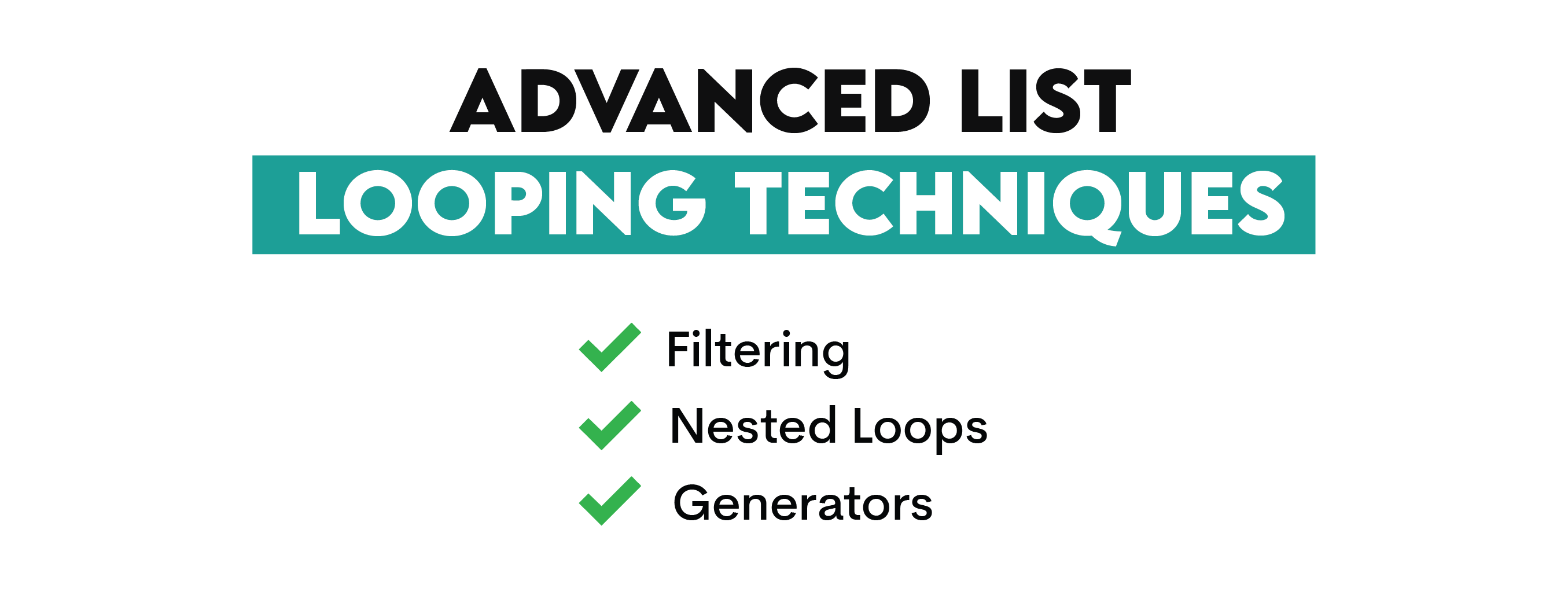
Filtering Elements While Looping
Want to know who earns the Option 1 bonus? Let’s filter:
- 8+ hours online
- 90%+ acceptance rate (after cleaning the %)
- 10+ trips
- A rating of 4.7 or better
Here is the code.
drivers = df.to_dict('records')
df['Accept Rate'] = df['Accept Rate'].astype(str).str.replace('%', '').astype(float)
qualified_option1 = [
d for d in drivers
if d['Supply Hours'] >= 8
and d['Accept Rate'] >= 90
and d['Trips Completed'] >= 10
and d['Rating'] >= 4.7
]
print(f"{len(qualified_option1)} drivers qualify for Option 1.")
Here is the output.

A sleek filtered list with only the cream of the crop. Just like that, you’ve got your bonus candidates.
Nested Loops
For example, imagine you want to compare each driver’s rating against every other driver’s rating. Weird use case? Maybe. But it’s a great excuse to demonstrate a nested loop in action.
Here is the code.
for i in range(len(drivers)):
for j in range(i + 1, len(drivers)):
diff = abs(drivers[i]['Rating'] - drivers[j]['Rating'])
if diff >= 3.4:
print(f"{drivers[i]['Name']} and {drivers[j]['Name']} differ in rating by {diff}")
Here is the output.

Nested loops are heavy. But when you're digging deep—like finding anomalies or rating clusters—they become necessary tools.
Using Generators for Large Lists
Generators are lazy — in a good way. They don’t eat memory. They don’t rush. They hand you item by item.
Let’s create a generator that only returns the names of drivers who completed fewer than five trips.
Here is the code.
def low_trip_driver_gen(data):
for d in data:
if d['Trips Completed'] < 5:
yield d['Name']
# Using the generator
for name in low_trip_driver_gen(drivers):
print(f"{name} did fewer than 5 trips")
Here is the output.

Instead of building a whole list, it hands you one result at a time. Perfect for big data or long pipelines.
Best Practices When Looping Through Lists
Looping seems manageable until it breaks something.
First, never change a list while looping it over. That’s a recipe for bugs. Always work with a copy (or create a new list to work from).
Second, when tracking positions or working with multiple lists, use tools such as `enumerate()` or `zip()`. They’re also more than fancy—they prevent index headaches.
And if your loop can be expressed in a single line, don’t overthink it. Python rewards silence and list comprehensions there for a reason.
Finally, when working with large data sets — imagine thousands of entries — generators will save you memory (and sanity).
Conclusion
Iterating through lists might seem like a noob skill — until you discover how central it is to real-world problem-solving.
Whether you’re filtering Uber drivers for payouts or analyzing rating gaps at scale, knowing when to loop makes your code tighter, faster, and easier to trust.
You’ve seen the basics. You’ve seen the edge cases. Now, it’s your turn to practice, play, and get your hands dirty.
If you enjoyed this breakdown, you’ll find plenty more like it on StrataScratch. You can jump straight into 700+ real data science interview questions and 50+ hands-on projects—ideal for rapid upskilling.
FAQs
What is the most efficient way to loop through a list in Python?
If you’re doing simple things, list comprehensions are often the fastest. They’re terse, readable, and Pythonic.
Can I loop through a list backward?
Yes. Python provides ways to change your loops' order without altering your original list. But it’s helpful when order matters or you’re unwinding something step by step.
fruits = ['apple', 'banana', 'cherry']
for fruit in reversed(fruits):
print(fruit)
How do I loop through a list of dictionaries?
You iterate over them as you would any list. These dictionaries act like a structured row, to which you can easily drill down to access fields by name, value, or ID.
for person in people:
print(f"{person['name']} is {person['age']} years old.")
Share