How to Perform Python String Concatenation?

Categories
Learn essential Python string concatenation techniques, including the + operator, join() method, f-strings, and format() method.
Have you ever thought about joining strings in Python? Understanding how to concatenate strings is vital to improving your programming efficiency and data manipulation techniques.
In this article, we will cover how to use string concatenation so you can see practical examples of combining them.
What is String Concatenation in Python?
Concatenation of strings is merging two or more strings into one. There are many ways to achieve it in Python, each with pros and cons and promising use cases.
These practices are essential for text generation data pre-processing for many projects involving natural language processing, data analysis, and formatting readable text in data science.
Let’s see a simple example.
# Define two strings
str1 = "Hello"
str2 = "World"
# Concatenate using the + operator
result = str1 + " " + str2
# Output the result
print(result)
Here is the output.

In this example, we use the + operator to join str1 and str2, with a space in between, resulting in the string "Hello World."
Why String Concatenation is Useful in Programming?
String concentration is helpful for many scenarios in Programming;
- Dynamic Messages: Concatenation builds custom messages for logging, user interfaces, and notifications.
- Data Manipulation: Concatenation is widely used in data science to clean and neaten data, merge columns, and create readable reports.
- File and URL generation: It works by concatenating strings to have dynamic file paths or URLs when working with files and the web, respectively.
Let’s see how concatenation works. In this example, we’ll see how it works to create a list of usernames and generate dynamic logging messages for each user.
# Define a list of usernames
usernames = ["Alice", "Bob", "Charlie"]
# Create dynamic messages using concatenation and a for loop
log_messages = ["User " + username + " has logged in." for username in usernames]
# Output each log message
for message in log_messages:
print(message)
Here is the output.

So, what we did here is use list comprehension and concatenate the static string User with the username and the string has logged in. This way, we optimize the generation of the dynamic messages list that we print using a for loop.
Basic String Concatenation Methods
There are several ways this is accomplished in Python. Both have various degrees of advantages and usage scenarios. Here are the most used methods:
- Concatenating with the + Operator: There is little explanation required, and it is simple to use for combining a few strings.
- Join () method: Preferred for Concatenating a huge number of strings or when strings are available as a list to concatenate more than one string.
Now, let's move on to each technique and provide an example.
+ Operator
The + operator is the simplest and most direct way to concatenate strings in Python. It is convenient but not extremely fast when faced with long lists of strings.
Here, we’ll use the + operator to combine multiple strings into one. Let’s see the code.
# Define multiple strings
str1 = "Data"
str2 = "Science"
str3 = "is"
str4 = "fun!"
# Concatenate using the + operator
result = str1 + " " + str2 + " " + str3 + " " + str4
# Output the result
print(result)
Here is the output.

It is straightforward. Let’s look at join() method.
join() Method
The join() method is faster than concatenating strings or lists of strings. It receives a sequence (such as a list) and concatenates its elements using a defined separator. Let’s see the code.
# Define a list of strings
words = ["Data", "Science", "is", "fun!"]
# Concatenate using the join() method
result = " ".join(words)
# Output the result
print(result)
Here is the output.

You can see here how we use the join() method to concatenate the list of strings, which is more efficient than using the + operator for large sequences.
Practical Example: Common Letters
Interview Question Date: February 2019
Find the top 3 most common letters across all the words from both the tables (ignore filename column). Output the letter along with the number of occurrences and order records in descending order based on the number of occurrences.
To illustrate both methods, let's solve a practical interview question. Here is the link to this question: https://platform.stratascratch.com/coding/9823-common-letters
The Problem Statement
Now, let’s break down this question into codeable steps.
Step 1: Preview
We have two different datasets. Let’s see them one by one, starting with google_file_store.
filename | contents |
---|---|
draft1.txt | The stock exchange predicts a bull market which would make many investors happy. |
draft2.txt | The stock exchange predicts a bull market which would make many investors happy, but analysts warn of possibility of too much optimism and that in fact we are awaiting a bear market. |
final.txt | The stock exchange predicts a bull market which would make many investors happy, but analysts warn of possibility of too much optimism and that in fact we are awaiting a bear market. As always predicting the future market is an uncertain game and all investors should follow their instincts and best practices. |
Here is the google_word_lists, our second dataset.
words1 | words2 |
---|---|
google,facebook,microsoft | flower,nature,sun |
sun,nature | google,apple |
beach,photo | facebook,green,orange |
flower,star | photo,sunglasses |
Step 2: Lowercase and Split Words
Now, let’s convert all words to lowercase and split them into individual words.
# Define a list of strings
words = ["Data", "Science", "is", "fun!"]
# Concatenate using the join() method
result = " ".join(words)
# Output the result
print(result)
Step 3: Concatenate Words
Combine all words into a single list.
# Concatenate Words
all_words = df1 + df2 + df3
Step 4: Join Words into a String
Use the join() method to create a single string from the list of words.
# Join Words into a String
tr = ' '.join(alist)
Step 5: Convert String to List of Characters
Convert the concatenated string into a list of characters.
# Convert String to List of Characters
a = list(tr)
Step 6: Create DataFrame and Clean the Data
Create a DataFrame from the list of characters and clean it by removing spaces.
# Create DataFrame and Clean Data
letters = pd.DataFrame(a, columns=['letter'])
letters['letter'].replace(' ', np.nan, inplace=True)
letters = letters.dropna()
Step 7: Count and Sort Letters
Count occurrences of each letter, sort them and get the top 3 most common letters.
# Count and Sort Letters
result = (letters.groupby('letter').size()
.to_frame('n_occurrences')
.reset_index()
.sort_values('n_occurrences', ascending=False)
.head(3))
Here is the entire code.
import pandas as pd
import numpy as np
# Step 2: Lowercase and Split Words
df1 = google_file_store.contents.str.lower().str.split(expand=True).stack().tolist()
df2 = google_word_lists.words1.str.split(',', expand=True).stack().tolist()
df3 = google_word_lists.words2.str.split(',', expand=True).stack().tolist()
# Step 3: Concatenate Words
alist = df1 + df2 + df3
# Step 4: Join Words into a String
tr = ' '.join(alist)
# Step 5: Convert String to List of Characters
a = list(tr)
# Step 6: Create DataFrame and Clean Data
letters = pd.DataFrame(a, columns=['letter'])
letters['letter'].replace(' ', np.nan, inplace=True)
letters = letters.dropna()
# Step 7: Count and Sort Letters
result = (letters.groupby('letter').size()
.to_frame('n_occurrences')
.reset_index()
.sort_values('n_occurrences', ascending=False)
.head(3))
Here is the output.
letter | n_occurences |
---|---|
a | 62 |
e | 53 |
t | 52 |
Advanced String Concatenation Techniques
In addition to the standard ways of simply adding some strings together, some extra options in Python give you more flexibility and efficiency.
Two Major Advanced Methods
F-strings: F-strings were introduced in Python 3.6, providing a simple way to evacuate expressions inside string literals.
Format () Method: This method was introduced for complex string formatting in Python and was meant to be an alternative to %-formatting.
Let’s discover them.
f-strings (Formatted String Literals)
f-strings are prefixed with 'f' and use curly braces “{}” to embed expressions inside string literals. They are concise and highly readable.
# Define variables
name = "Alice"
age = 30
scores = [85, 92, 78]
average_score = sum(scores) / len(scores)
# Concatenate using f-strings
report = (
f"Student Name: {name}\n"
f"Age: {age}\n"
f"Scores: {scores}\n"
f"Average Score: {average_score:.2f}\n"
f"Status: {'Passed' if average_score > 80 else 'Failed'}"
)
# Output the result
print(report)
Here is the output.
This example shows how using f-strings allows us to inject the name and age variables directly into the string.
format() Method
Format() method is used to format strings by using curly braces {} as placeholders that will be replaced with the values you pass into the format() method.
We’ll use it to create a detailed table for product information. Here is the code.
products = [
{"name": "Laptop", "price": 1200, "quantity": 5},
{"name": "Smartphone", "price": 800, "quantity": 10},
{"name": "Tablet", "price": 300, "quantity": 15}
]
# Construct table header
table_header = "{:<15} {:<10} {:<10}".format("Product", "Price", "Quantity")
print(table_header)
print("-" * 35)
# Construct table rows
for product in products:
table_row = "{:<15} ${:<9} {:<10}".format(product["name"], product["price"], product["quantity"])
print(table_row)
Here is the output.
In this example, we created a layout using the format() method and displaying information from products about the product name, price, and quantity of the products.
If you want to discover more methods, check out Python String Methods.
Practical Examples
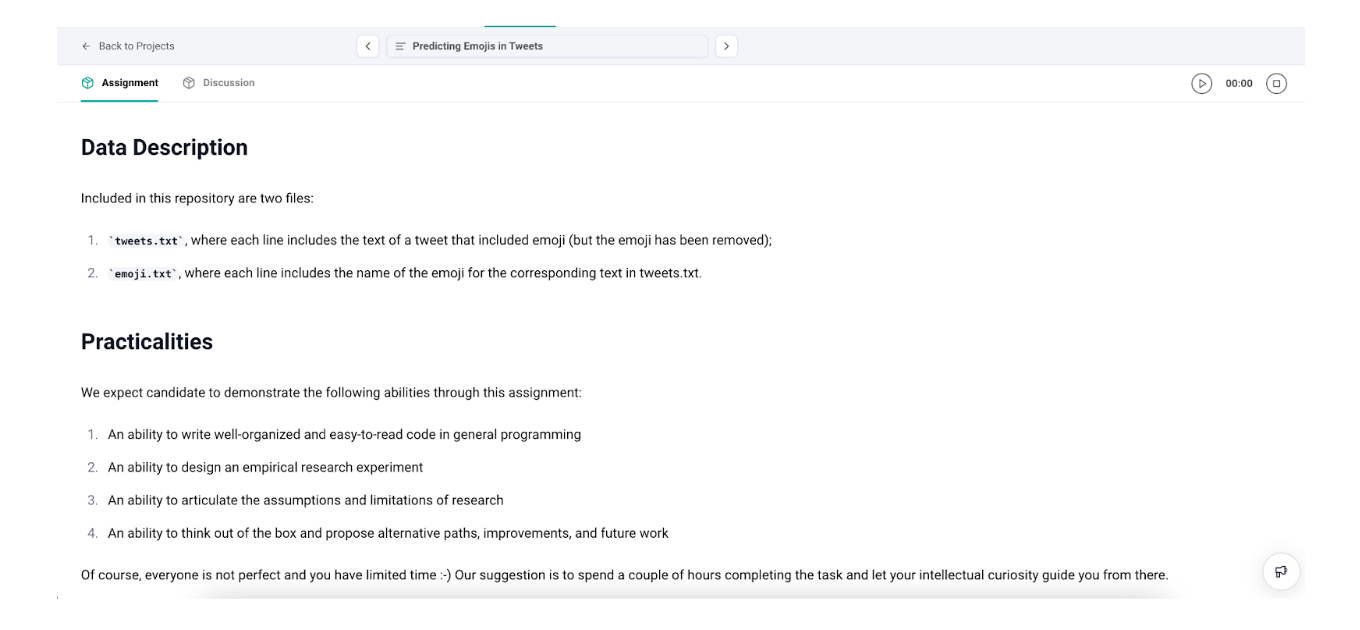
String concatenation is helpful in various real-world scenarios, particularly in data science, web development, and automation tasks.
This section will explore how string concatenation can be applied in different practical scenarios by using the Predicting Emojis in Tweets data project.
Project Description
This data project has been used as a take-home assignment in the Emogi data science recruiting process. The objective is to construct a natural language model that will relate a sequence of words.
- Tweets.txt: It contains a line per tweet with emojis in the tweet text, with the emojis strippedannotation_mecab_generative_tweets.strip_emoji.
- emoji.txt with a line containing the name of molecule emoji for text at the same line in the tweets.txt.
Creating a Word Cloud
A word cloud is a sophisticated and visual representation of information based on text data. The size of individual words shows the count or relevance in the given text data.
Word cloud visualization software is applied to preprocess and concatenate all the text data into one string. The following example shows how to concatenate the strings to prepare the text data for creating a word cloud.
Let’s visualize tweets. Here is the code.
# Import necessary libraries
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# Concatenate all tweets into a single string
all_tweets = ' '.join(tweets_df['tweet'].tolist())
# Generate a word cloud
wordcloud = WordCloud(width=800, height=400, background_color='white').generate(all_tweets)
# Display the word cloud using matplotlib
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
Here is the output.
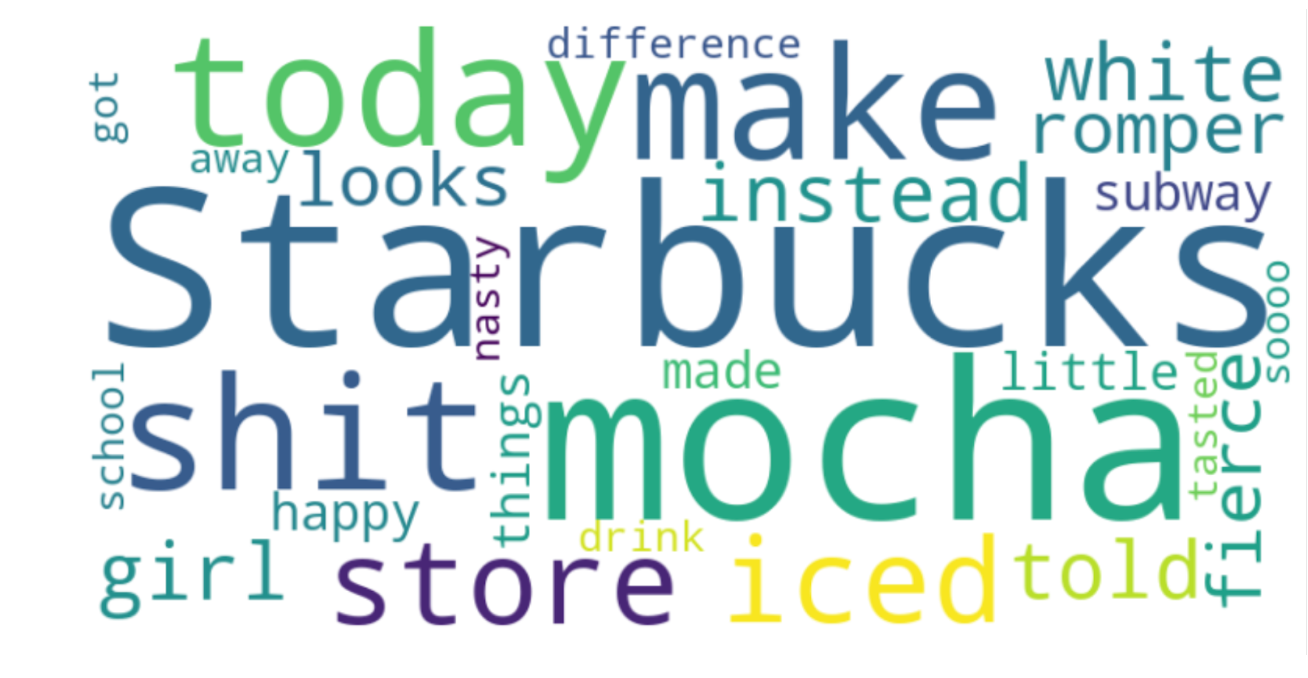
Logging and Debugging Messages
In this part, we can add a debugging display for the tweet text and respective emojis. This shows we are pairing the dataset properly.
Let’s see the code.
# Create a list to hold log messages
log_messages = []
# Generate log messages from the dataset
for tweet, emoji in zip(tweets_df['tweet'], emojis_df['emoji']):
log_message = f"Tweet: {tweet} | Emoji: {emoji}"
log_messages.append(log_message)
# Output the first 10 log messages
for message in log_messages[:10]:
print(message)
Here is the output.

Why did we use f-strings here?
- Data Verification: Make sure every tweet is associated with the right emoji.
- Debugging: Identify when data looks wrong or contains errors
- Readable: With f-strings, log messages are clear and legible.
- Logger: To keep logs that are easily readable with data validation information.
This example shows why it is good to concatenate more strings if you need them to create a log message that tells you something more during data validation (and the debugging).
Tips for Choosing the Right Method Based on Performance Needs
Choosing the right way to string concatenate is important because it can make your code faster or more readable. Some tips that will help you decide on which one to use in different scenarios:
- Number of Strings
- Small number of strings: When the string count is too low, using the + operator is simple. It is great for small concatenation jobs.
- Large number of strings: If there are many strings to be concatenated, especially in a loop, then use the join() method. This method saves us from creating many interim string objects.
- Readable/Readable Editable
- f-strings: They are formatted string literals that are more readable and concise than string.format(). This allows expressions to be embedded directly in the string. F-strings--when you need to include values or expressions in a neat and more readable way.
- The format() Method: It offers a more generic way to format strings, especially for concerns that are more complex for our needs or do not work on versions of Python less than 3.6.
- Performance Considerations
- Complexity: The join() method is usually faster than concatenation because it accomplishes the concatenation in a single pass. This can be especially useful in applications that rely on performance.
- Memory: For large strings, using the join() method reduces memory usage and prevents the creation of all intermediate big string objects!***.
- Compatibility
- Python Version: If you need to run your code on a Python version older than 3.6, default to the.format() method rather than f-strings. This makes it compatible with many Python environments.
- Complex Formatting
- f-strings and format() Method - f-strings and the format() method offer comprehensive formatting options such as specifying number formats, aligning text, etc. Pick the method that best fits how you want to format code and provides the fairest opportunity for readability.
Before finalizing this one, check out these Python Interview Questions.
Conclusion
We covered the + operator, join() method, f-strings, and the format() method, each suited for different tasks and needs.
Choosing the correct method improves code performance and readability, essential for efficient programming.
Practice these techniques with examples and experiment on StrataScratch to enhance your skills.
