How to Get the Index of an Item in a List in Python
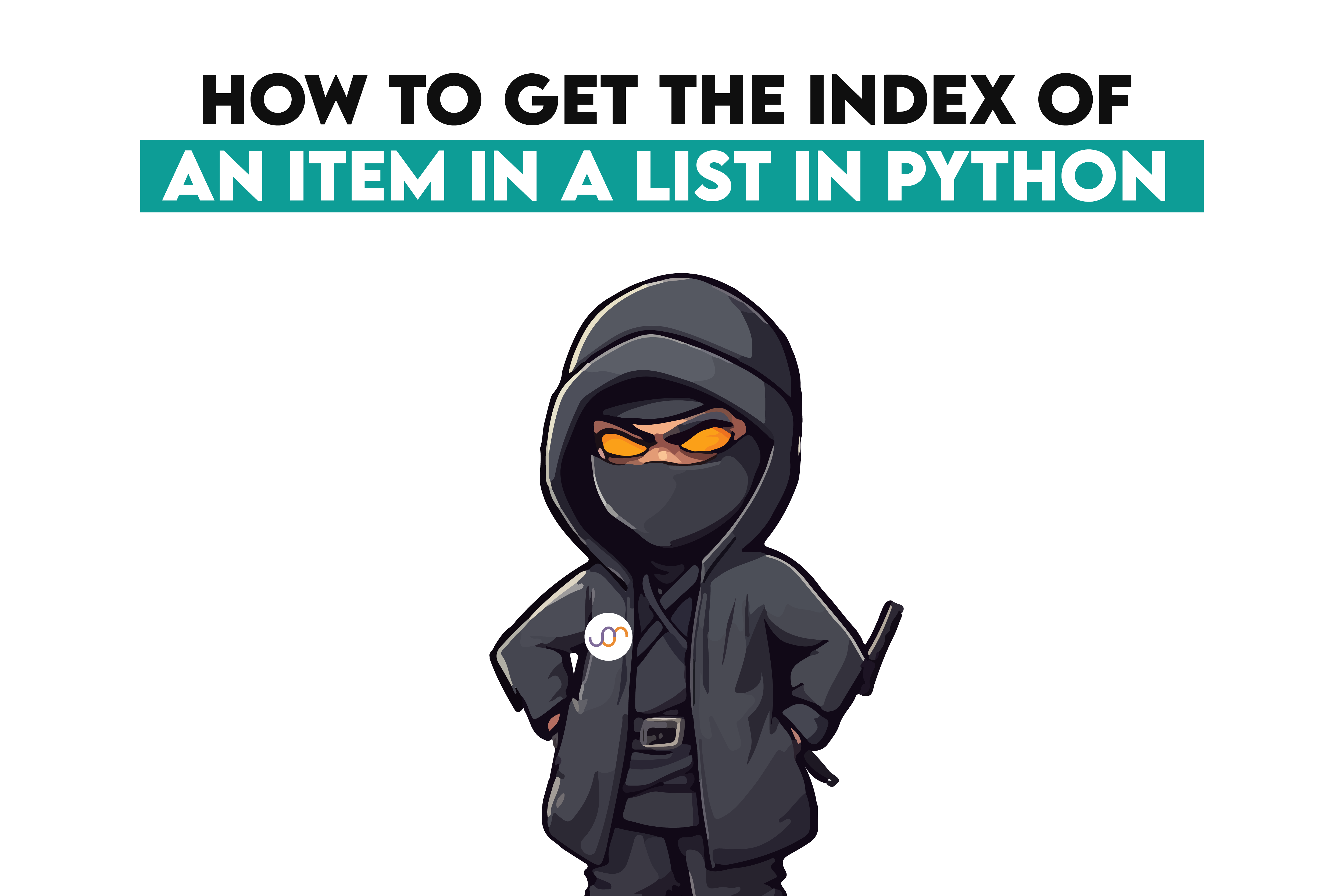
- Written by:
Nathan Rosidi
Master Python's index() function to efficiently locate items in lists, handle errors with custom functions, and improve data analysis using advanced techniques.
A Python list can contain not one but multiple types of data in one place. Exactly the programmatic flexibility makes lists a cornerstone resource in the geeks of all guises’ toolbox.
That is why, in this article, we’ll guide you through the simplicity of finding items in lists by using Python’s index() tool and discover some less basic techniques the function can master for you in more customized circumstances.
What is a List in Python?
A list in Python is a very useful container that can hold different types of items, such as numbers, strings, or even other lists. It works like a container of other elements, a flexible box allowing you to group several items. A list is a collection of ordered items where an index can access each item.
This applies especially to data science, where you must handle and apply operations on your dataframe or set. Lists are, hence, an essential ingredient of your Python toolkit.
ages = [25, 30, 22, 26, 32]
first_age = ages[0]
print(first_age)
Here is the output.

In this list, the first age, 25, is at index 0, the second age, 30, is at index 1, and so on.
After sorting, the index position tells which age is at what place in the list. For example, 25 comes first with an index value of 0, and the second with the lowest value will make you get my point right.
Syntax of List index()
A function called index tells you the position of an item in a list. It searches the list and returns the index of the item to be found first.
It is a straightforward yet effective way to know its proper location in your data.
list_name.index(item)
The index () value gives the position in the list where the item exists. If it is not, Python will kick a ValueError.
Applied Example:
So, pretend you have a list of temperatures recorded over the week and want to know when it reached 30 degrees for the first time.
temperatures = [28, 29, 30, 32, 31, 30, 29]
first_30_index = temperatures.index(30)
print(first_30_index)
Here is the output.

This will return 2, indicating that 30°C was first recorded at index 2.
How to Find the Index of an Item in a List in Python
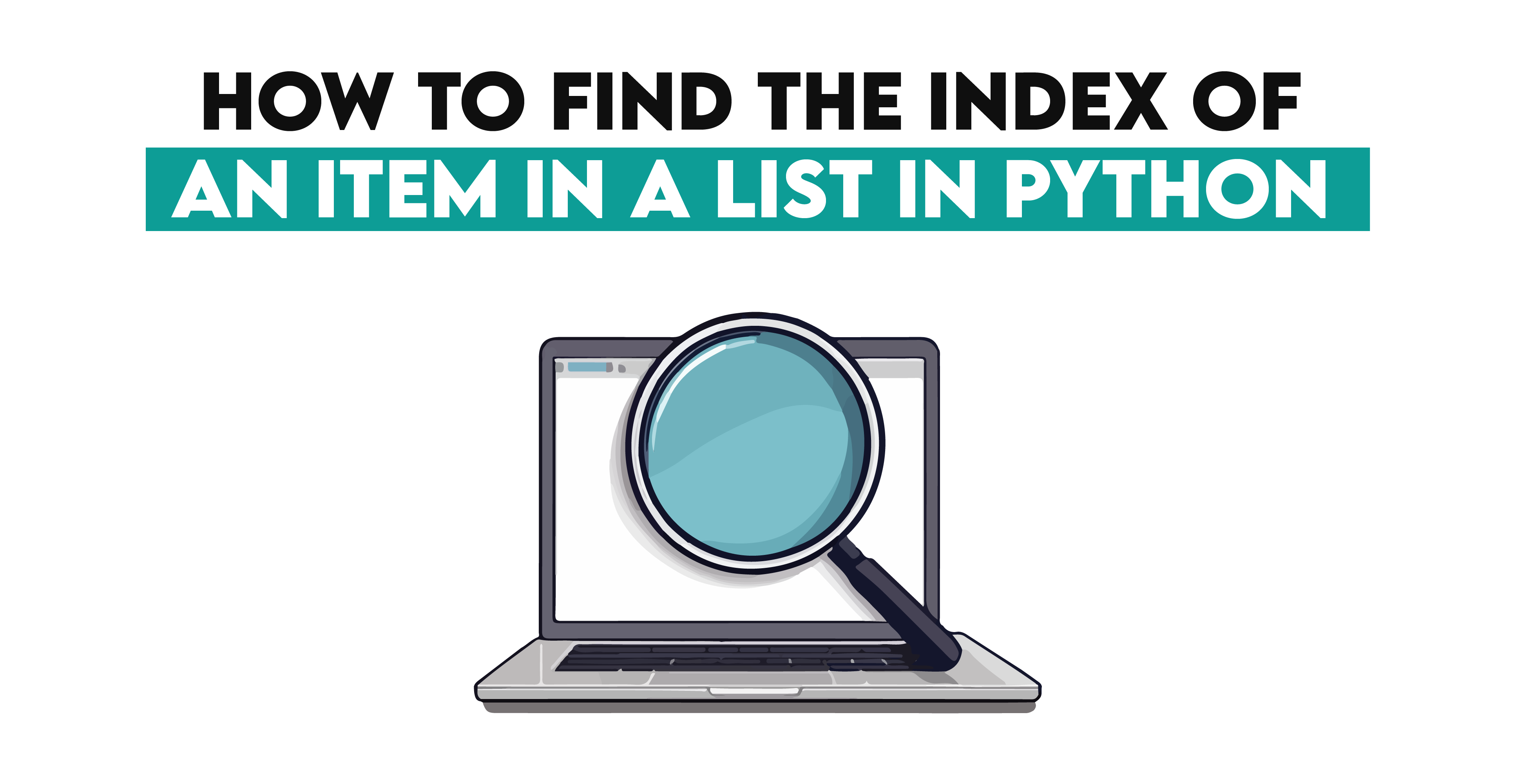
Index() will merely get you an option for the position of an element in a list, and the title "the best" one is often used between coders.
Let´s say that, in some cases, you must control how we find when an item does not exist in the list, or we want to locate all occurrences of this element, not only the first.
Custom Approach with Error Handling:
Imagine you have a list of employee IDs and need to find an ID. You return a custom message instead of allowing your code to crash when the ID is absent.
employee_ids = ['E001', 'E002', 'E003', 'E004', 'E005']
def find_index(item, lst):
try:
return lst.index(item)
except ValueError:
return f"Item {item} not found in the list"
result = find_index('E003', employee_ids)
print(result)
result_not_found = find_index('E007', employee_ids)
print(result_not_found)
Here is the output.

In the above code, we created a user-defined function find_index() and wrapped it in Python’s index(). The function tries to find the index of the item that you specified. It returns the index if successful and catches a ValueError to exit with a custom message instead.
This is especially helpful when dealing with large datasets that may be missing data, simply because checking null for every piece of column value access will make your code base much more error-prone.
Basic Method: Using the index() Function
The index() method is a simple way to find an item's position in a list. It is best suited for searches over sorted collections because its approach makes it a safe bet when you know an item should be on the list.
You may need to change your strategy if you are working with more complex data structures (e.g., a list in another list). For example, suppose your dataset is a time series in which each entry records an event at some specific datetime location. In that case, you can use the index() method to infer when that particular event occurred.
event_log = [
'Start_Process',
'Load_Data',
'Error_404',
'Retry_Connection',
'Success',
'End_Process'
]
error_index = event_log.index('Error_404')
print(f"'Error_404' first occurred at position: {error_index}")
Here is the output.

This is where the index() comes in handy. It allows you to find the first event that occurs and is very valuable, especially when analyzing sequences with event ordering.
If you want to know more, check out our post “Python List Methods”.
Handling Exceptions
The index () function will always return the position in a list as declared, but there are chances where it may not exist. Otherwise, Python raises a ValueError. However, it will break your program if it is not handled correctly.
Advanced Example with Exception Handling:
Consider you are reading sensor readings and want to get the first occurrence of a specific type of reading. You should try catching it here so the program will not crash and produce an error if no such reading exists.
sensor_readings = [50, 55, 60, 65, 70]
def find_reading_index(reading, readings):
try:
return readings.index(reading)
except ValueError:
return "Reading not found in the list"
result = find_reading_index(65, sensor_readings)
print(result)
result_not_found = find_reading_index(75, sensor_readings)
print(result_not_found)
Here is the output.

This approach makes your program tolerant to unavailable data by returning a custom message and does not break the code due to missing expected values.
Finding Indices of Duplicate Items
Sometimes, you will be required to find the first appearance of an item in a list and all places where it appears. This is a common data-cleaning scenario because we expect survey responses to have duplicates (but different responses recorded once). In repeated measures studies, there will be duplicate measurements.
The index() function in Python returns only the first occurrence; therefore, you need a friend to return all indices where an item shows up.
Using a List Comprehension:
An example of how to find all occurrences of an element in an efficient manner is through list comprehension. This way, you can loop through the list and keep collecting on which index your item appears.
Example:
Let's say you have a list of survey results and want to find all the places where some results, such as "Yes," are present.
responses = ['Yes', 'No', 'Yes', 'Maybe', 'Yes', 'No']
yes_indices = [i for i, response in enumerate(responses) if response == 'Yes']
print(yes_indices)
Here is the output.

Here, the list comprehension is externally iterating and going through each of the responses. This is because the enumerate() function gives both index and item, so we must check if the item is "Yes." The index is in the list of yes_indices. If so
This is especially handy if you intend to investigate patterns or occurrences of a given value throughout the data because it shows all instances.
Custom Function to Find Index
Sometimes, using the built-in index() function needs to be more flexible. For instance, you could search for an item only under some conditions, or there are situations when more than one criterion detects the index you're looking for. Creating a function that searches for the index can be your savior in these cases.
Creating a Custom Function:
Imagine you have a list of dictionaries — here, each dictionary corresponds to a record in your dataset. It could be that you have to find the index of the first record that meets a condition but with logical OR (e.g., specific value in one field and range of values in another)
Example:
Imagine you have a list of employee records, with some fields plus the years they worked. Then, you need to find an employee who is an engineer and has worked in that position for more than five years.
employees = [
{'name': 'Alice', 'title': 'Engineer', 'years_experience': 4},
{'name': 'Bob', 'title': 'Engineer', 'years_experience': 6},
{'name': 'Charlie', 'title': 'Manager', 'years_experience': 10},
{'name': 'David', 'title': 'Engineer', 'years_experience': 3},
]
def find_employee_index(employees, title, min_experience):
for i, employee in enumerate(employees):
if employee['title'] == title and employee['years_experience'] > min_experience:
return i
return "No matching employee found"
index = find_employee_index(employees, 'Engineer', 5)
print(index)
Here is the output.

This is a custom function find_employee_index() that iterates over the list of employee records. It evaluates all the records by a condition that they have to be Title belongs to the employee_type AND (Sumii+Exp+Coding_Experience >= Threshold). The function then returns the index of that employee if both conditions are satisfied. It returns a custom message if no employee meets the criteria.
This strategy comes in handy when your criteria for a search are more sophisticated than just an item by its value. This is important since you have many facets influencing an answer in the real world.
Using List Comprehensions for Efficiency
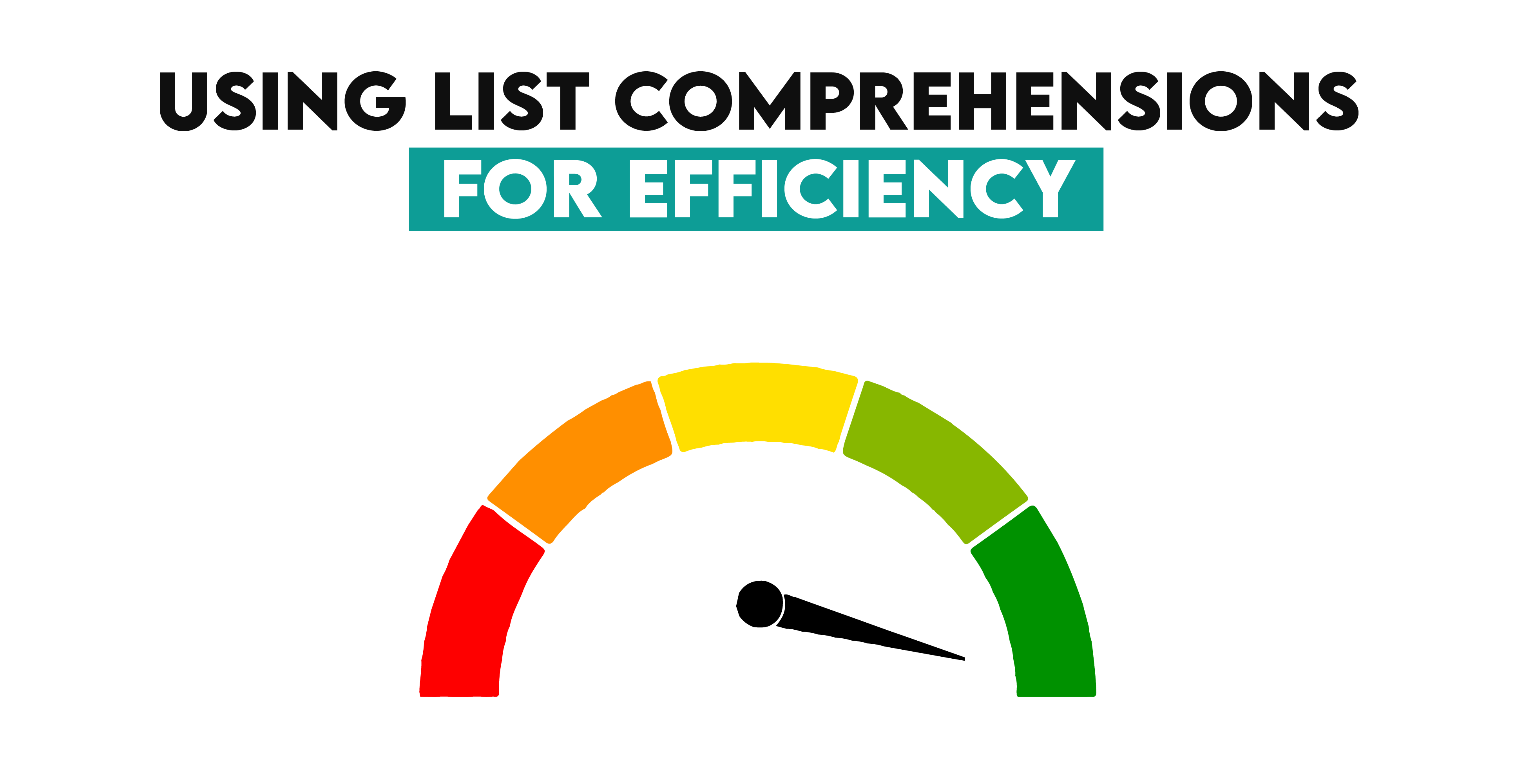
In Python, list comprehension is a feature that allows you to write new lists that are simpler and readable by iterating over existing ones. List comprehension is most commonly used for either filtering data or performing some transformation on a subset of your dataset based upon certain criteria.
With list comprehensions, you can search for an item in a list and apply compound conditions to get what you want.
Why List Comprehensions?
List comprehensions are not only more succinct than using traditional loops, as above, but they are also typically faster.
This is especially useful while dealing with large bulky datasets or when you are paranoid about performance.
List comprehensions are handy for everything and anything, especially when working with data (in my experience), which is the best way.
Example:
For example, you have a dataset that records the daily temperature in Celsius for the last month and returns all days on which the temperature was above 30 degrees.
daily_temperatures = [28, 32, 29, 35, 33, 30, 31, 36, 29, 28, 32, 31, 34, 29, 30, 28, 27, 32, 30, 34, 35, 33, 29, 30, 31, 30, 34, 36, 37, 29]
hot_days_indices = [i for i, temp in enumerate(daily_temperatures) if temp > 30]
print(hot_days_indices)
Here is the output.

In this example, the list comprehension iterates through a list and accesses each array.
This is a very efficient and concise way to quickly determine whether certain days were especially hot without using more logic in this loop.
Practical Applications: Real-world Scenarios
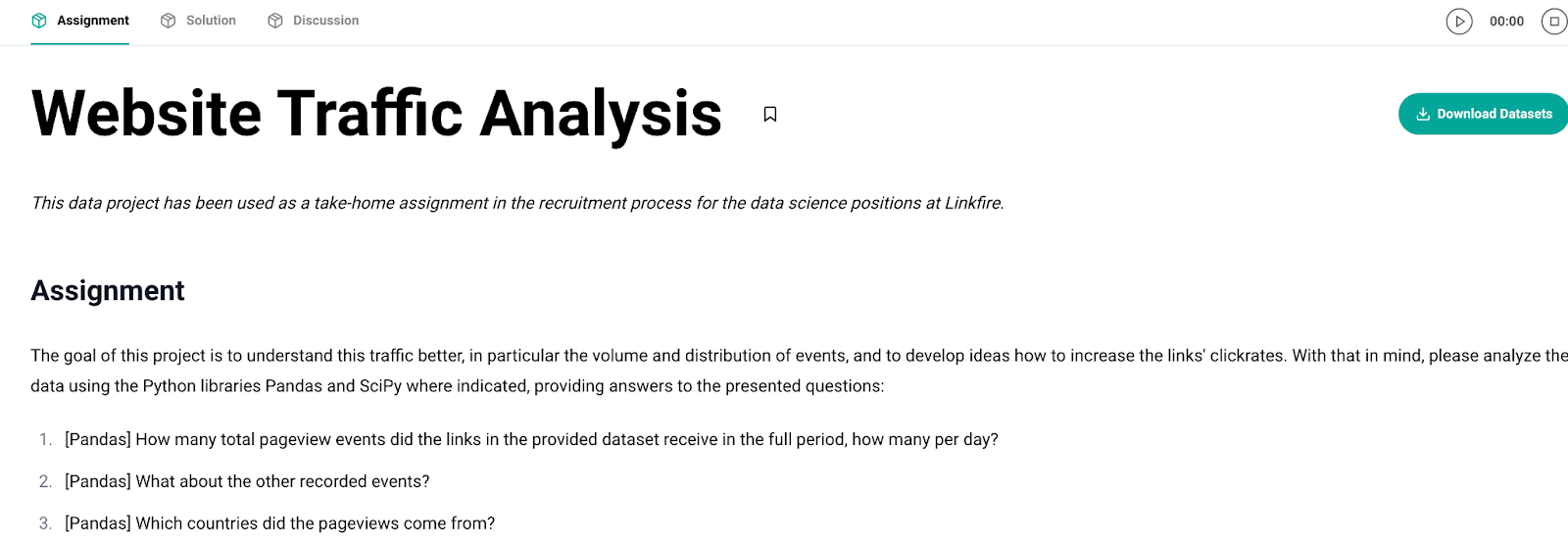
Linkfire requested an analysis of web traffic data. The use case is to understand the load of traffic by looking at the volume and spread of events and build strategies to improve CTR on links.
Link to this project: https://platform.stratascratch.com/data-projects/website-traffic-analysis
Step 1: Load and Explore the Dataset
First, we load the dataset to understand its structure:
import pandas as pd
file_path = 'traffic.csv'
traffic_data = pd.read_csv(file_path)
print(traffic_data.head())
Here is the output.
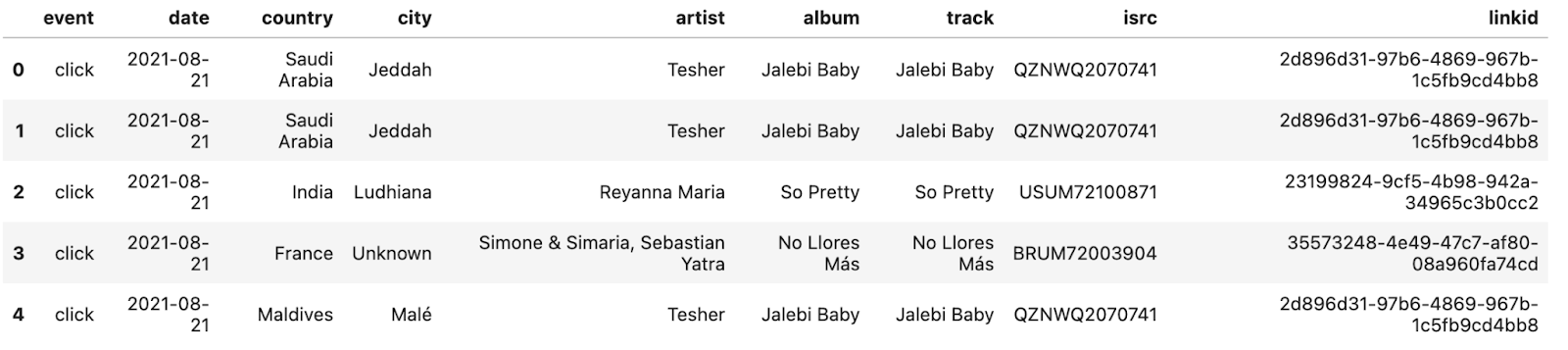
Step 2: Using index() to Find Specific Link Data
Let's say you want to find the index of a specific link in your dataset—one with an ISRC value, for instance—and extract its metrics.
target_isrc = 'USUM72100871'
link_index = traffic_data.index[traffic_data['isrc'] == target_isrc].tolist()
if link_index:
print(f"Index of link with ISRC {target_isrc}: {link_index[0]}")
else:
print(f"Link with ISRC {target_isrc} not found in the dataset.")
Here is the output.

Step 3: Counting Specific Events for a Link
We first want to bucket and count all click events between a particular (it's just an ID) link based on its ISRC value.
target_isrc = 'BRUM72003904'
specific_link_data = traffic_data[(traffic_data['isrc'] == target_isrc) & (traffic_data['event'] == 'click')]
clicks_count = specific_link_data.shape[0]
print(f"Total clicks for the link with ISRC {target_isrc}: {clicks_count}")
pageviews_data = traffic_data[(traffic_data['isrc'] == target_isrc) & (traffic_data['event'] == 'pageview')]
pageviews_count = pageviews_data.shape[0]
if pageviews_count > 0:
click_rate = clicks_count / pageviews_count
print(f"Click Rate for the link with ISRC {target_isrc}: {click_rate:.2%}")
else:
print(f"No pageviews recorded for the link with ISRC {target_isrc}")
Here is the output.

Conclusion
Here, we have explored how to efficiently find where data may be using the index() function. We have also executed other techniques for analyzing those specific data points in more simple lists and complex datasets, such as website traffic.
Finally, we’ve covered the basics of lists, the use of the index () function, and how best to use custom approaches and list comprehensions for efficient data handling.
Learn from a variety of datasets with scenarios. Better yet, try out various functions to become a pro in data analysis with Data Projects like we did in this article.
Share