How to Determine Python String Length?
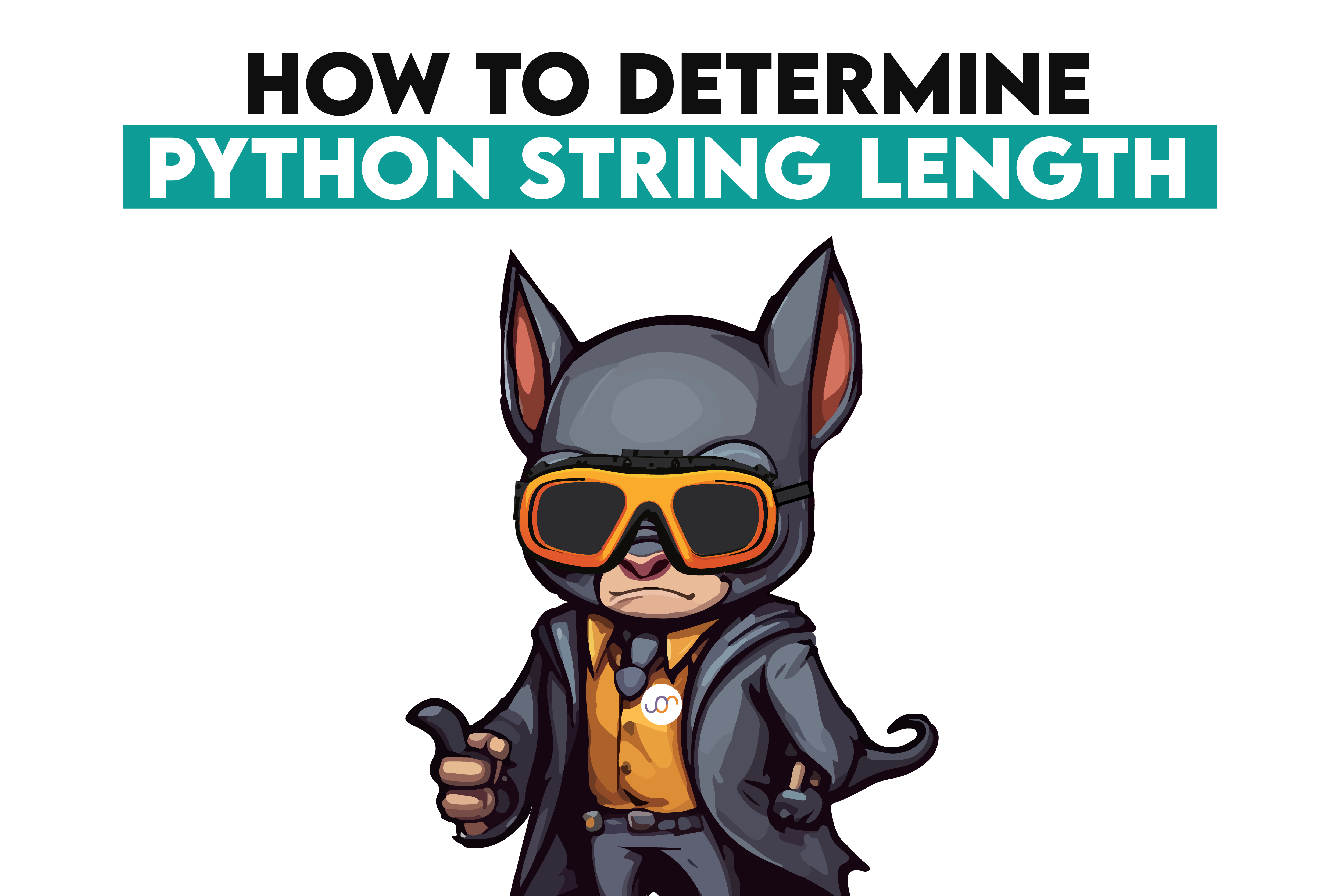
Categories
Discover the simple yet powerful tools Python offers to measure string length effortlessly, enhancing your coding efficiency and data manipulation skills.
Have you ever scratched your head and wondered how to measure a string in Python? You're not alone! Many folks think it's a Herculean task requiring complex coding gymnastics. But guess what? Python's got a secret weapon up its sleeve.
Buckle up because I'm about to unveil the surprisingly simple world of string length in Python.
We'll discover Python's nifty tools that make string measuring a breeze. By the end, you'll count characters like a pro, so no sweat! Let’s start.
Basic Properties of Python Strings
Do you know the basic properties of Python strings? They are not just character sequences. You can use them for text manipulation, and if you are interested in Data Science, make sure you will do a lot!
That’s why learning the fundamentals of Python string should be very important. So, let’s start with its fundamentals first.
Immutability
Python strings resist change like stubborn mules. Why? Because, once they were created, they stay put. Why does this matter to you?
Because consistency becomes your best friend in your coding adventures. Immutability ensures changes don’t ripple unexpectedly. Let’s see one quick example!
original_string = "Hello World!"
modified_string = original_string.replace("World" "Python")
print("Original:" original_string)
print("Modified:" modified_string)
Here is the output.

Notice how original_string remains unaltered? That's immutability at work, folks!
Indexing and Slicing
Python lets you play surgeon with strings. To do so, you have to know Indexing. It helps you pinpoint specific characters while slicing carves out substrings.
Slicing will allow you to divide strings into characters by selecting them. Let's see indexing and slicing in action:
string = "Data Science"
first_character = string[0]
last_character = string[-1]
substring = string[5:12]
print("First Character:", first_character)
print("Last Character:", last_character)
print("Substring:", substring)
Here is the output.

You've just dissected "Data Science" like a pro! First character? 'D'. The last one? 'e'. And "Science" emerges from slicing.
Remember when you struggled with text parsing? These techniques would've saved you hours of headache. Next time you're knee-deep in data, try applying indexing and slicing. You might surprise yourself with its efficiency.
Tip: Experiment with negative indices for some mind-bending string manipulation. They count backward from the string's end!
The len() Function to Find String Length in Python
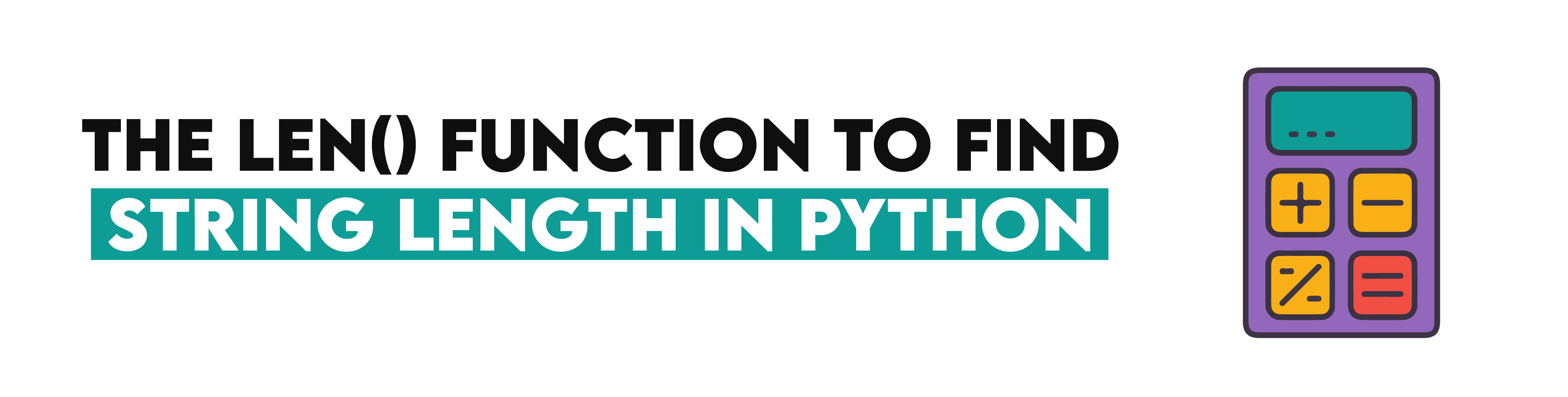
Let’s say you want to measure the length of the strings in Python, but how? Meet len(), your new best friend, for string measurements. This nifty built-in function makes counting characters easier.
Syntax and Usage
Using len() is simple. Just toss your string into it, and voila! You get a number back. Let’s test it!
Use Case: Find the number of open businesses
Find the number of open businesses.
In this question, yelp wants us to find the number of open businesses. Here is the link to the question : https://platform.stratascratch.com/coding/10051-find-the-number-of-open-businesses/
Here is our dataset.
business_id | name | neighborhood | address | city | state | postal_code | latitude | longitude | stars | review_count | is_open | categories |
---|---|---|---|---|---|---|---|---|---|---|---|---|
G5ERFWvPfHy7IDAUYlWL2A | All Colors Mobile Bumper Repair | 7137 N 28th Ave | Phoenix | AZ | 85051 | 33.45 | -112.07 | 1 | 4 | 1 | Auto Detailing;Automotive | |
0jDvRJS-z9zdMgOUXgr6rA | Sunfare | 811 W Deer Valley Rd | Phoenix | AZ | 85027 | 33.68 | -112.08 | 5 | 27 | 1 | Personal Chefs;Food;Gluten-Free;Food Delivery Services;Event Planning & Services;Restaurants | |
6HmDqeNNZtHMK0t2glF_gg | Dry Clean Vegas | Southeast | 2550 Windmill Ln, Ste 100 | Las Vegas | NV | 89123 | 36.04 | -115.12 | 1 | 4 | 1 | Dry Cleaning & Laundry;Laundry Services;Local Services;Dry Cleaning |
pbt3SBcEmxCfZPdnmU9tNA | The Cuyahoga Room | 740 Munroe Falls Ave | Cuyahoga Falls | OH | 44221 | 41.14 | -81.47 | 1 | 3 | 0 | Wedding Planning;Caterers;Event Planning & Services;Venues & Event Spaces | |
CX8pfLn7Bk9o2-8yDMp_2w | The UPS Store | 4815 E Carefree Hwy, Ste 108 | Cave Creek | AZ | 85331 | 33.8 | -111.98 | 1.5 | 5 | 1 | Notaries;Printing Services;Local Services;Shipping Centers |
As you can see, al we have to do is;
- Filter the open restaurants
- Count the number of open restaurants
Here is the code.
import pandas as pd
import numpy as np
is_open = yelp_business[yelp_business['is_open'] == 1]
result = len(is_open)
Here is the output.
Boom! You've just counted 78 restaurants under 1 second.
Performance Considerations
You might worry about len() slowing things down with massive strings. Fear not! This function's got speed to spare. It runs lightning-fast, no matter how long your string gets.
Picture this: You've got a ginormous log file. Millions of characters. Scary right? Not for len()! Let’s test it!
log_file = "Error: Oops!\n" * 1000000 # One beefy log file
length_of_log = len(log_file)
print("Log file length:" length_of_log)
Here is the output.

Zippy as ever, len() crunches through that monster file without breaking a sweat. Are you impressed yet?
Use Case: Find the number of Yelp businesses that sell pizza
Find the number of Yelp businesses that sell pizza.
In this problem, yelp wants to analyze the dataset and find several Yelp businesses that sell pizza. Here is the question’s link: https://platform.stratascratch.com/coding/10153-find-the-number-of-yelp-businesses-that-sell-pizza
Here are the dataset’s first rows.
business_id | name | neighborhood | address | city | state | postal_code | latitude | longitude | stars | review_count | is_open | categories |
---|---|---|---|---|---|---|---|---|---|---|---|---|
G5ERFWvPfHy7IDAUYlWL2A | All Colors Mobile Bumper Repair | 7137 N 28th Ave | Phoenix | AZ | 85051 | 33.45 | -112.07 | 1 | 4 | 1 | Auto Detailing;Automotive | |
0jDvRJS-z9zdMgOUXgr6rA | Sunfare | 811 W Deer Valley Rd | Phoenix | AZ | 85027 | 33.68 | -112.08 | 5 | 27 | 1 | Personal Chefs;Food;Gluten-Free;Food Delivery Services;Event Planning & Services;Restaurants | |
6HmDqeNNZtHMK0t2glF_gg | Dry Clean Vegas | Southeast | 2550 Windmill Ln, Ste 100 | Las Vegas | NV | 89123 | 36.04 | -115.12 | 1 | 4 | 1 | Dry Cleaning & Laundry;Laundry Services;Local Services;Dry Cleaning |
pbt3SBcEmxCfZPdnmU9tNA | The Cuyahoga Room | 740 Munroe Falls Ave | Cuyahoga Falls | OH | 44221 | 41.14 | -81.47 | 1 | 3 | 0 | Wedding Planning;Caterers;Event Planning & Services;Venues & Event Spaces | |
CX8pfLn7Bk9o2-8yDMp_2w | The UPS Store | 4815 E Carefree Hwy, Ste 108 | Cave Creek | AZ | 85331 | 33.8 | -111.98 | 1.5 | 5 | 1 | Notaries;Printing Services;Local Services;Shipping Centers |
To find businesses that sell Pizza, we need only filter categories and find the businesses that include the “Pizza” keyword in the categories. Here is the code to find these businesses;
import pandas as pd
import numpy as np
pizza = yelp_business[yelp_business['categories'].str.contains('Pizza', case = False)]
Here is the output.
business_id | name | neighborhood | address | city | state | postal_code | latitude | longitude | stars | review_count | is_open | categories |
---|---|---|---|---|---|---|---|---|---|---|---|---|
dRb2Xq8jorJV6tDCgmaQUg | Papa John's Pizza | 703 E Bell Rd | Phoenix | AZ | 85022 | 33.64 | -112.07 | 5 | 3 | 1 | Restaurants;Pizza | |
MYB1ZMspBk1Xc_awp_PtSw | Naked City Pizza Express | Southwest | 6935 Blue Diamond Rd | Las Vegas | NV | 89178 | 36.02 | -115.24 | 3 | 46 | 1 | Sandwiches;Chicken Wings;Restaurants;Pizza |
XVDR44P_74FmA0ANanm4CQ | House of Pizza | Plaza Midwood | 3640 Central Ave | Charlotte | NC | 28205 | 35.22 | -80.78 | 3.5 | 75 | 0 | Greek;Restaurants;Pizza;Italian |
CV05rBOr5DdDGvxUZkRFmg | Angeline's | Uptown | 303 S Church St | Charlotte | NC | 28202 | 35.23 | -80.85 | 3.5 | 17 | 1 | Restaurants;Nightlife;Pizza;Cocktail Bars;Bars;Italian |
EsE8KTPqAJ2MjJdmuAifRw | Dante's Inferno Flats | East Bank | 1059 Old River Rd | Cleveland | OH | 44113 | 41.5 | -81.71 | 3.5 | 21 | 1 | Italian;Restaurants;Pizza |
Now that you’ve found the businesses, you should count their number. To do that, we can use our len() function. Here is the code.
Include the “Pizza” keyword in the categories. Here is the code to find these businesses;
import pandas as pd
import numpy as np
pizza = yelp_business[yelp_business['categories'].str.contains('Pizza', case = False)]
result = len(pizza)
Here is the output
As you can see, we can find the total number of businesses that sell pizza without the len() function.
Using Loops to Determine String Length in Python
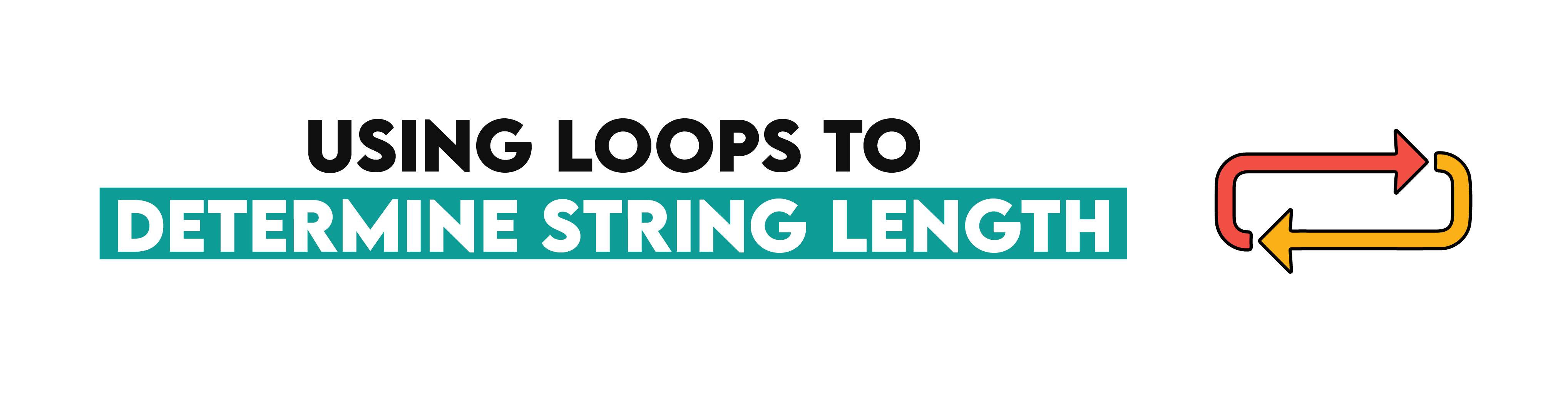
Sure, len() rocks, but what if you want to flex your coding muscles? Loops offer a hands-on approach to string measuring. Let's go into these loop-de-loops!
For Loops
Let’s say you're crafting a custom string processor. Suddenly, you need to count characters manually. Enter for loop your trusty sidekick! Here is the code;
string = "Data Science"
length_of_string = 0
for char in string:
length_of_string += 1
print("String length (for loop):" length_of_string)
Here is the output.

You've just counted 12 characters one by one.
While Loops
Now, let's shake things up with a while loop. Why? Because sometimes you need more control over your character-counting shenanigans.
Let’s say you're hunting for a specific character. A while loop lets you call shots as you go.
string = "Data Science"
length_of_string = 0
index = 0
while index < len(string):
length_of_string += 1
index += 1
print("String length (while loop):", length_of_string)
Here's the output

Look at that! Another way to reach 12. You're on a roll!
Tip: While loops shine when you need conditional exit points. Do you have a unique character to stop at? While loops got your back.
Handling Unicode and Special Characters
In this section, we will analyze text, including special characters like foreign languages and emojis.
Unicode Strings
Unicode: the superhero of character encoding! It swoops in to save multilingual texts from digital oblivion. Python embraces this champion wholeheartedly.
Let’s say you have global user feedback from different countries, and you have to analyze these texts. Daunting, right? Now, with Python! Let's whip up a linguistic smoothie. Here is the code.
english_text = "Hello"
korean_text = "안녕하세요"
emoji_text = "😊"
combined_text = english_text + " " + korean_text + " " + emoji_text
print("Multilingual mix:", combined_text)
print("Character count:", len(combined_text))
Here is the output.

Voila! English, Korean, and emoji all coexist peacefully. Python counts each character flawlessly regardless of its origin.
Encoding and Decoding
Now, let's tackle encoding and decoding. It is like translating between human speech and computer gibberish.
Let’s say you must save a file with exotic characters. Encoding rushes in as your digital translator. Let’s see;
original_text = "안녕하세요 😊"
encoded_text = original_text.encode('utf-8')
decoded_text = encoded_text.decode('utf-8')
print("Human-readable:" ,original_text)
print("Computer-gibberish:", encoded_text)
print("Back to human:", decoded_text)
Here is the output.

Ta-da! Your text just journeyed through computer-land and returned unscathed.
Tip: Always specify your encoding when working with files. 'utf-8' usually does the trick, but know your data's origin!
Practical Applications of Python String Length
Now, let’s look at two practical applications of Python string length manipulation: data validation and text analysis.
Data Validation
Let’s say you are building a web form where users must pick usernames, but you can’t let them go wild. Here, you can enter Python string length, which will validate the usernames. Let’s see!
def validate_username(username):
min_length = 5
max_length = 15
if len(username) < min_length:
return "Too short! Try again."
elif len(username) > max_length:
return "Whoa there! Too long."
else:
return "Perfect! You're good to go."
Now, let’s test this code.
username = "DataNinja"
validation_result = validate_username(username)
print("Verdict:", validation_result)
Here is the output.

Boom! You've just created a digital bouncer for usernames. No riffraff allowed!
Text Analysis
Here, let’s write a function that will measure the length of the given strength. You can use this feature in combination with anything. For example, you can set a limit on your website that will not allow users to add comments above any given length.
Let’s see our sentence-length analyzer.
import re
def average_sentence_length(text):
sentences = re.split(r'[.!?]' text)
sentence_lengths = [len(sentence.strip()) for sentence in sentences if sentence.strip()]
if not sentence_lengths:
return 0
return sum(sentence_lengths) / len(sentence_lengths)
text = "Data science rocks! It makes sense of chaos. Python rules the data world."
avg_length = average_sentence_length(text)
print("Average sentence length:" avg_length)
Here is the output.

Look at that! You've just x-rayed a text snippet measuring its linguistic bones.
Tip: Combine sentence length analysis with readability scores for deeper insights into text complexity.
Common Errors and Troubleshooting
To avoid common errors and prevent them before happening, you can add additional steps of codes.
TypeError Handling
Let’s say you're zipping through a list, processing items left and right. Suddenly bam! A wild TypeError appears.
What should you do? Here, you can implement a try and except block to avoid this error and add the error code you want. Here is the code.
items = ["Hello", "World”, 123, "Python"]
for item in items:
try:
length = len(item)
print(f"'{item}' is {length} characters long")
except TypeError:
print(f"Oops! Can't measure '{item}'. It's not a string!")
Here is the output.

Look at that! You've just built a safety net for your code. No more crashing when non-strings crash the party.
Conclusion
In this article, we first explored the len() function with loops and different kinds of characters. Next, we’ve explored practical applications like data validation and text analysis.
These techniques, methods, and applications enhance your skills in string manipulation and make you read for different types of scenarios.
If you want to explore more practical examples, check out “Python String Methods” and learn how to effectively clean and format strings. Practice these methods, by using different python interview questions and data projects from our platform.
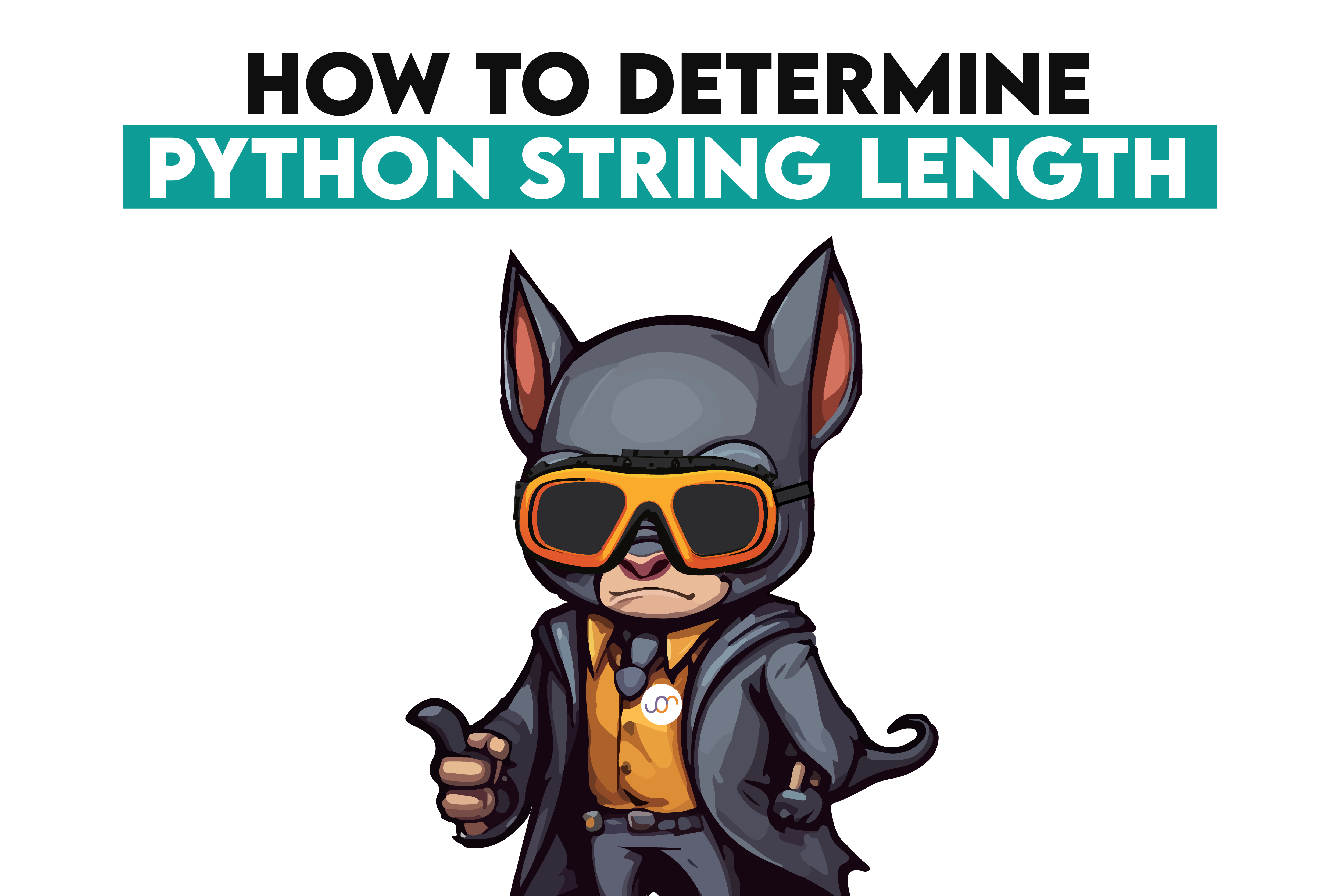