From Arrays to Lists: Converting Numpy Arrays with Python
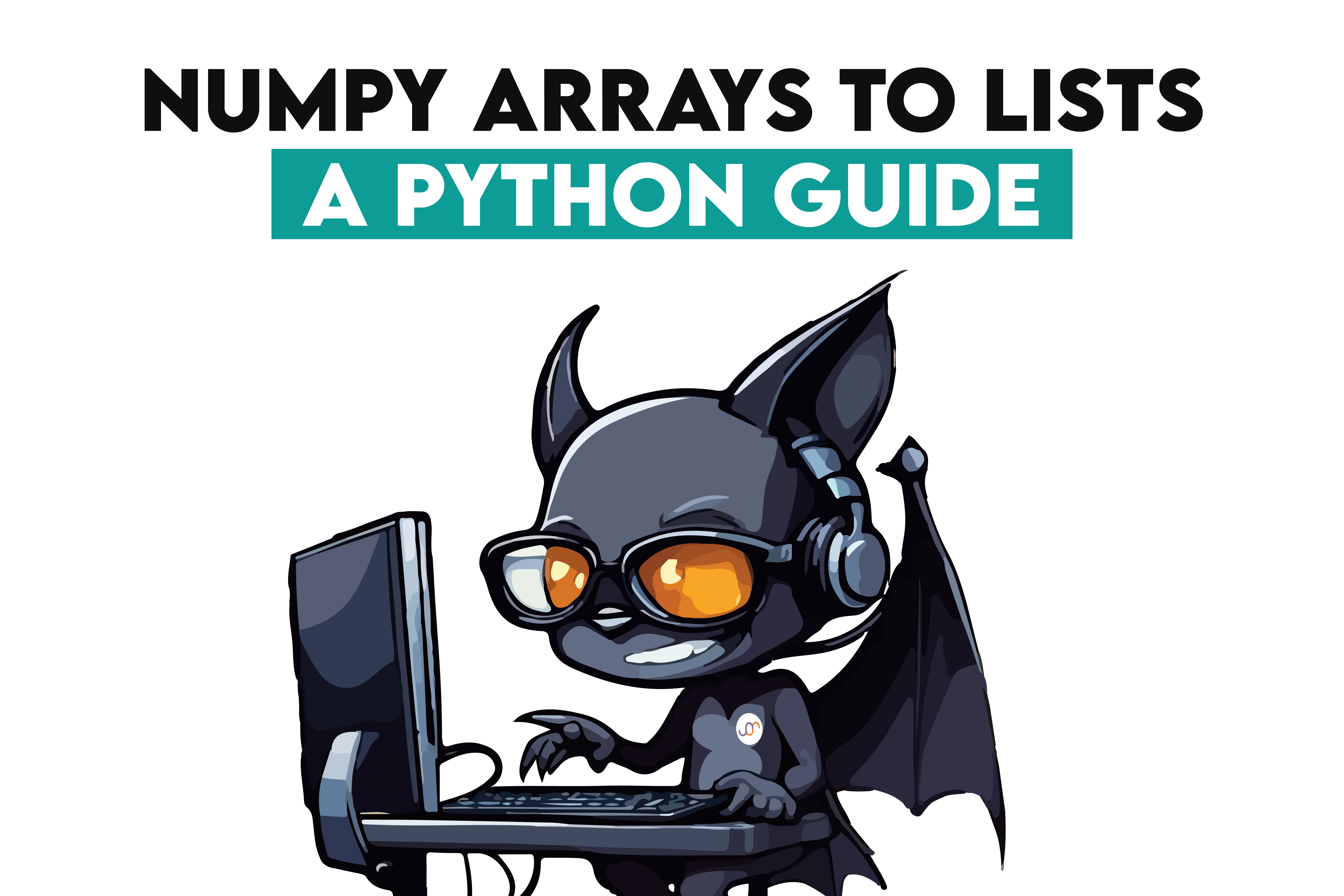
Categories:
- Written by:
Nathan Rosidi
Learn the essential methods for converting NumPy arrays to Python lists, enhancing your data handling skills in Python with practical examples and applications.
Have you ever faced challenges switching between different data structures in Python? As a data scientist knowing how to convert Numpy arrays into Python lists should be in your toolbox.
In this article, you’ll learn many simple and effective methods for making these conversions, with many examples. So open your Jupyter notebook or visit our platform to do examples while reading this one. Let’s get started!
Understanding NumPy Arrays
Numpy stands for "Numerical Python." It supports large multidimensional arrays and matrices and provides various mathematical functions to operate on these arrays. Its efficiency and ease of use make Numpy a valuable data science toolkit.
Common Use Cases of NumPy Arrays
Numpy arrays are primarily used in data science tasks because they are functional structures. They enable streamlined processing and analysis, from basic statistical operations to machine learning algorithms.
They’re also used in data cleaning, transformation, simulations, and many other situations, which we will cover in this one.
Creating NumPy Arrays
But let’s start with the basics and see how it can be created. We will see four different methods of creating NumPy arrays. Let’s start with the first method.
Using np.array
With this function, you can convert a list or a tuple into an array. So, if you have a tuple and list to create an array, this must be your choice. Let’s see how it can be used.
import numpy as np
array_from_list = np.array([1, 4, 7, 14, 15])
print("Array from list:", array_from_list)
Here is the output.

Now, this time, let’s turn a tuple to the array.
# Creating an array from a tuple
array_from_tuple = np.array((1, 4, 7, 14, 15))
print("Array from tuple:", array_from_tuple)
Here is the output.

As you can see, the outputs are the same, but let’s use NumPy’s other cool feature to see if these arrays are equal. Here is the code.
arrays_are_equal = np.array_equal(array_from_list, array_from_tuple)
print("Are the arrays equal?", arrays_are_equal)
Here is the output.

As you can see from this example, NumPy can create arrays from tuples and lists. But what about other options?
Using np.arange
With the arange() method from numpy, you can generate arrays in sequential order by giving them a limit.
Let’s say you want to create an array that starts from 0 and goes to 9; here is the code.
array_one_to_ten = np.arange(10)
array_one_to_ten
Here is the output.

Let’s say you want to see the odd numbers between one and thirty-one. Here, we’ll define the range and the step size.
Here is the code.
array_odds = np.arange(1, 32, 2)
array_odds
Here is the output.

As you can see, in the second number, we did not write 31, instead we did write 32.
Now that you know how to create an array, let’s look at other methods for creating a numpy array filled with zeros and ones. Also, if you want to know more about NumPy methods, check this “NumPy for Data Science Interviews”.
Using np.zeros
Let’s create a numpy array filled with zeros. Here is the code.
array_zeros = np.zeros(5)
array_zeros
Here is the output.

Using np.ones
This time, let’s create a numpy array filled with ones. Here is the code.
array_ones = np.ones(5)
array_ones
Here is the output.

This one allows you to see more complex arrays, such as 2D or 3D arrays filled with ones or zeros.
array_zeros_2d = np.zeros((2, 3))
print(array_zeros_2d)
array_ones_2d = np.ones((2, 3))
print(array_ones_2d)
Here is the output.
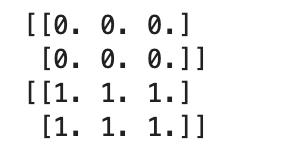
Do you want to know how to select elements of an array by using slicing methods? Check this “NumPy Array Slicing in Python”.
Understanding Python Lists
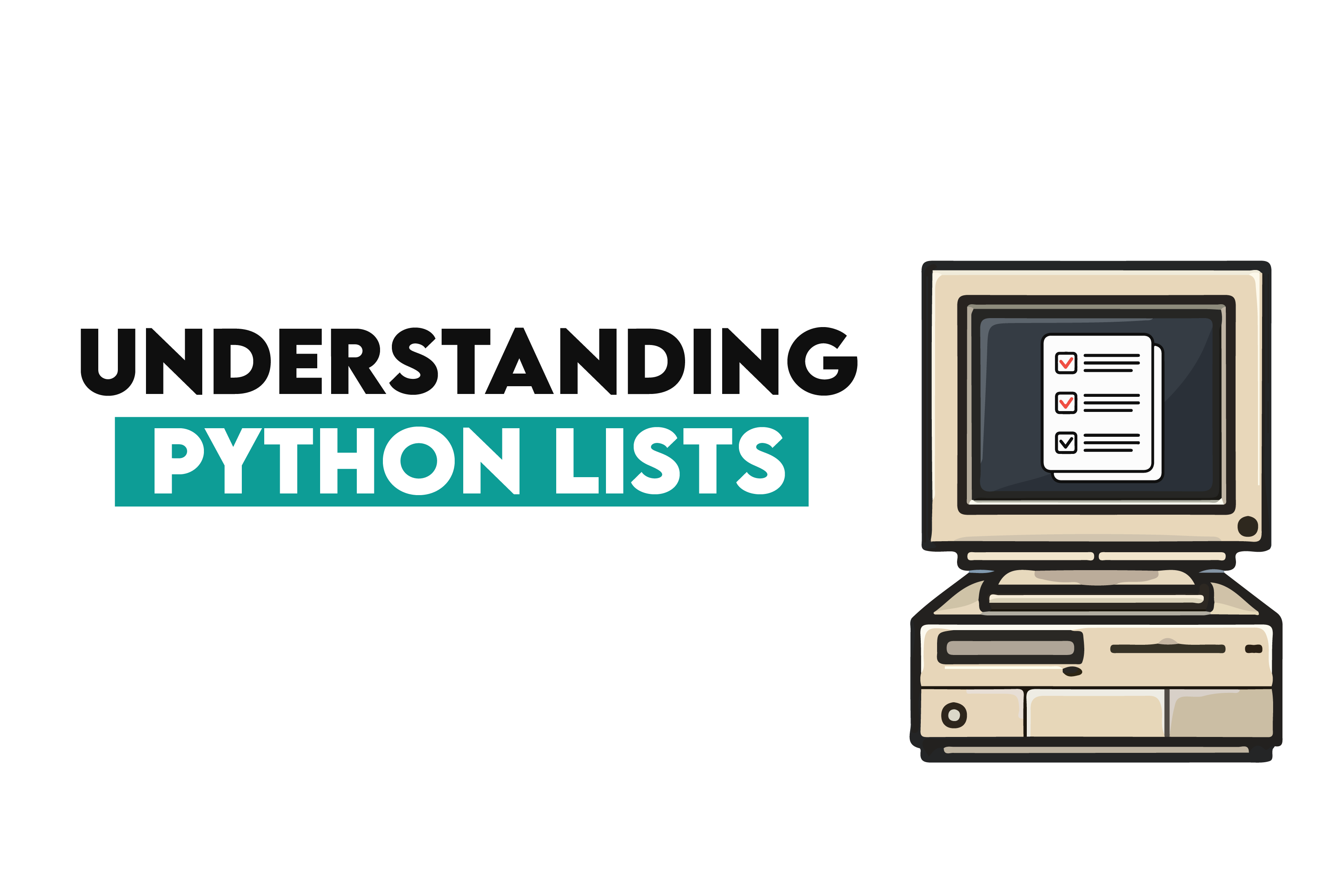
Python lists are one of the fundamental data structures used extensively in more complex constructs to store collections of items. They are also operator-friendly, making them great for data science and more.
Definition and Importance
A Python list can include more than one type. Unlike arrays, lists are mutable. What does it mean? It means you can change their content without creating a whole new one. This will make Python lists helpful in handling dynamic data.
Common Use Cases of Python Lists
Python lists are used to store collections. This could include storing numbers, names, or lists of lists. Data processing, web development, data science, and other dynamic applications require lists.
Let’s see its characteristics.
Characteristics of Python Lists
Python lists have several key characteristics that make them useful:
- Mutable: Contents can be changeable
- Dynamic Sizing: Size can change dynamically.
- Heterogeneous Elements: They can contain elements of different types.
Properties of Lists
Lists are mutable and can be changed after creation. For example, create a list filled with numbers and words and change the 3rd element of it.
However, be careful; Python is a zero-indexed language, meaning the index of the first element is 0, so to change the third element, you must use my_list[2]. Let’s see the code.
my_list = [1, 5, "A" , 12, 25]
print(my_list)
my_list[2] = 10
print(my_list)
Here is the output.

We created a list in this code and then changed the 3rd element.
Adding, Removing, and Modifying Elements
Adding elements to a list in Python can be done using the following methods;
- append()
- extend(),
- insert().
Here, we will see an example using append(), extend(), and insert() to add multiple elements in different positions within the list. Let’s see the code.
my_list = [1, 2, 3, 4, 5]
my_list.append(6)
print(f"After append: {my_list}")
additional_elements = [7, 8, 9]
my_list.extend(additional_elements)
print(f"After extend: {my_list}")
positions_and_elements = [(1, 'A'), (4, 'B'), (7, 'C')]
for pos, elem in positions_and_elements:
my_list.insert(pos, elem)
print(f"After multiple inserts: {my_list}")
Here is the output.

What have we done here? First, we added a single element with append and multiple with extend. Next, we added elements to the specified positions using the insert method.
Let’s see methods for removing elements from the list.
Removing Elements from a List
Removing elements from a list can be done using methods like;
- remove()
- pop()
- Del
Below, we will show these methods, including removing elements by index and value. Let’s see the code.
# Initial list
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 'A', 'B', 'C']
my_list.remove(2)
print(f"After remove(2): {my_list}")
removed_element = my_list.pop(3)
print(f"After pop(3) (removed {removed_element}): {my_list}")
indices_to_remove = [0, 2, 5]
for index in sorted(indices_to_remove, reverse=True):
del my_list[index]
print(f"After multiple deletions: {my_list}")
Here is the output.

Here, we first remove elements using the remove-and-pop method. Then, we use the del method to remove multiple elements from the list.
Finding Mutual Friends Using Lists and Pandas
Last Updated: January 2024
You are analyzing a social network dataset at Google. Your task is to find mutual friends between two users, Karl and Hans. There is only one user named Karl and one named Hans in the dataset.
The output should contain 'user_id' and 'user_name' columns.
To showcase another practical use case of lists, here is an interview question from Google: https://platform.stratascratch.com/coding/10365-common-friends-script
Google wants you to analyze a social network dataset. Your task is to find mutual friends between two users, Karl and Hans.
To solve this problem, we should follow the steps below;
- Finding User IDs
- Finding Friends of Karl and Hans:
- Finding Mutual Friends' IDs
- Getting Mutual Friends' User Names
Here is the solution.
import pandas as pd
# Find the user IDs for Karl and Hans
karl_id = users[users['user_name'] == 'Karl']['user_id'].iloc[0]
hans_id = users[users['user_name'] == 'Hans']['user_id'].iloc[0]
# Find friends of Karl and Hans
karl_friends = friends[friends['user_id'] == karl_id]['friend_id'].tolist()
hans_friends = friends[friends['user_id'] == hans_id]['friend_id'].tolist()
# Find mutual friends' IDs
mutual_friends_ids = list(set(karl_friends).intersection(hans_friends))
# Get mutual friends' user names
mutual_friends = users[users['user_id'].isin(mutual_friends_ids)]
mutual_friends
Here is the output.
In the code above, we found mutual friends between Karl and Hands by taking advantage of the list operations. This shows how lists can be combined with dataframes to analyze and process data.
Converting Numpy Arrays to Python Lists
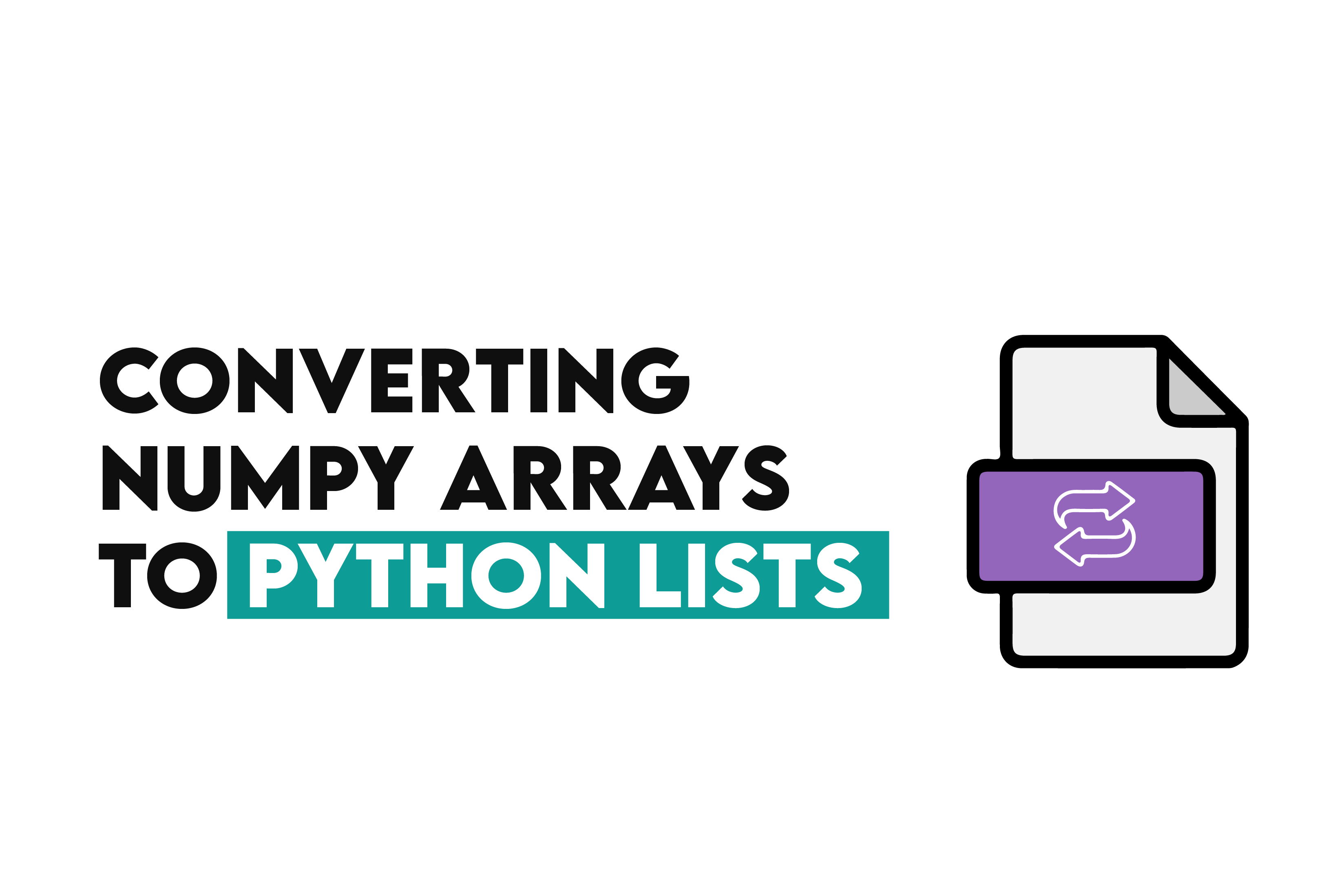
The tolist() method in Numpy provides a straightforward way to convert a Numpy array into a Python list. This method is simple and direct, ensuring that all elements in the Numpy array are transformed into a list format. Let’s see with an example.
Here, we define an array and turn it into a list using the tolist() method.
Here is the code.
import numpy as np
array = np.array([1, 2, 3, 4, 5])
list_from_array = array.tolist()
print(list_from_array)
Here is the output.

For a complex example, now let’s convert a 2D Numpy array into a nested list. Here is the code.
array_2d = np.array([[1, 2, 3], [4, 5, 6]])
list_from_array_2d = array_2d.tolist()
print(list_from_array_2d)
Here is the output.

Alternative Methods of Conversion
While tolist() is the most straightforward method, there are alternative methods to convert Numpy arrays to Python lists, like list comprehension. So, let’s test it.
import numpy as np
array = np.array([1, 2, 3, 4, 5])
list_from_array_comprehension = [x for x in array]
print(list_from_array_comprehension)
Here is the output.

Here, we create an array and convert it to a list using list comprehension.
Other Methods to Convert Numpy Arrays to Lists
Also, you can create an empty list and add elements inside it using a for loop. These methods are not straightforward, but they offer different approaches that can be useful in various scenarios.
Let’s see the code.
import numpy as np
array = np.array([1, 2, 3, 4, 5])
list_from_array_loop = []
for element in array:
list_from_array_loop.append(element)
print(list_from_array_loop)
Here is the output.

As you can see, multiple methods exist to convert numpy arrays to python lists. If you want to know more about Python Lists, check this comprehensive guide to Python Lists.
Practical Applications and Examples
Converting NumPy arrays to Python lists can be important in data analysis. When? Especially if you prefer working with libraries that prefer lists or selecting lists because they might be faster to process.
Let’s explore a practical example from our platform. This example aims to predict delivery duration. In the first section, we will analyze the dataset, and in the second subsection, we will build a model that predicts delivery duration.
Use case in Data Analysis
First, let’s load the dataset, process the data, and use Numpy arrays and Python lists for some data manipulations. We will show converting DataFrame columns to Numpy arrays and lists to perform necessary operations.
import pandas as pd
import numpy as np
file_path = 'historical_data.csv'
data = pd.read_csv(file_path)
created_at_array = pd.to_datetime(data['created_at']).to_numpy()
actual_delivery_time_array = pd.to_datetime(data['actual_delivery_time']).to_numpy()
delivery_duration_seconds = (actual_delivery_time_array - created_at_array).astype('timedelta64[s]').astype(int)
delivery_duration_list = delivery_duration_seconds.tolist()
data['delivery_duration_seconds'] = delivery_duration_list
data.head()
Here is the output.

After evaluating the output, we calculated the delivery duration in seconds and added it to the dataset. This process involved converting DataFrame columns to Numpy arrays and then to lists for further manipulation.
Final Thoughts
In this article, we’ve explored how to convert NumPy arrays to lists. First, we explored these data structures and saw their use cases.
Switching between these data structures will increase your ability to handle several tasks in Python and give you confidence working with different data structures.
First, create arrays and convert them into lists using our platform to improve your data handling capabilities. For further examples, practice with real-life related datasets and explore different methods to find the best for you.
Share