Adding and Removing List Elements with Python
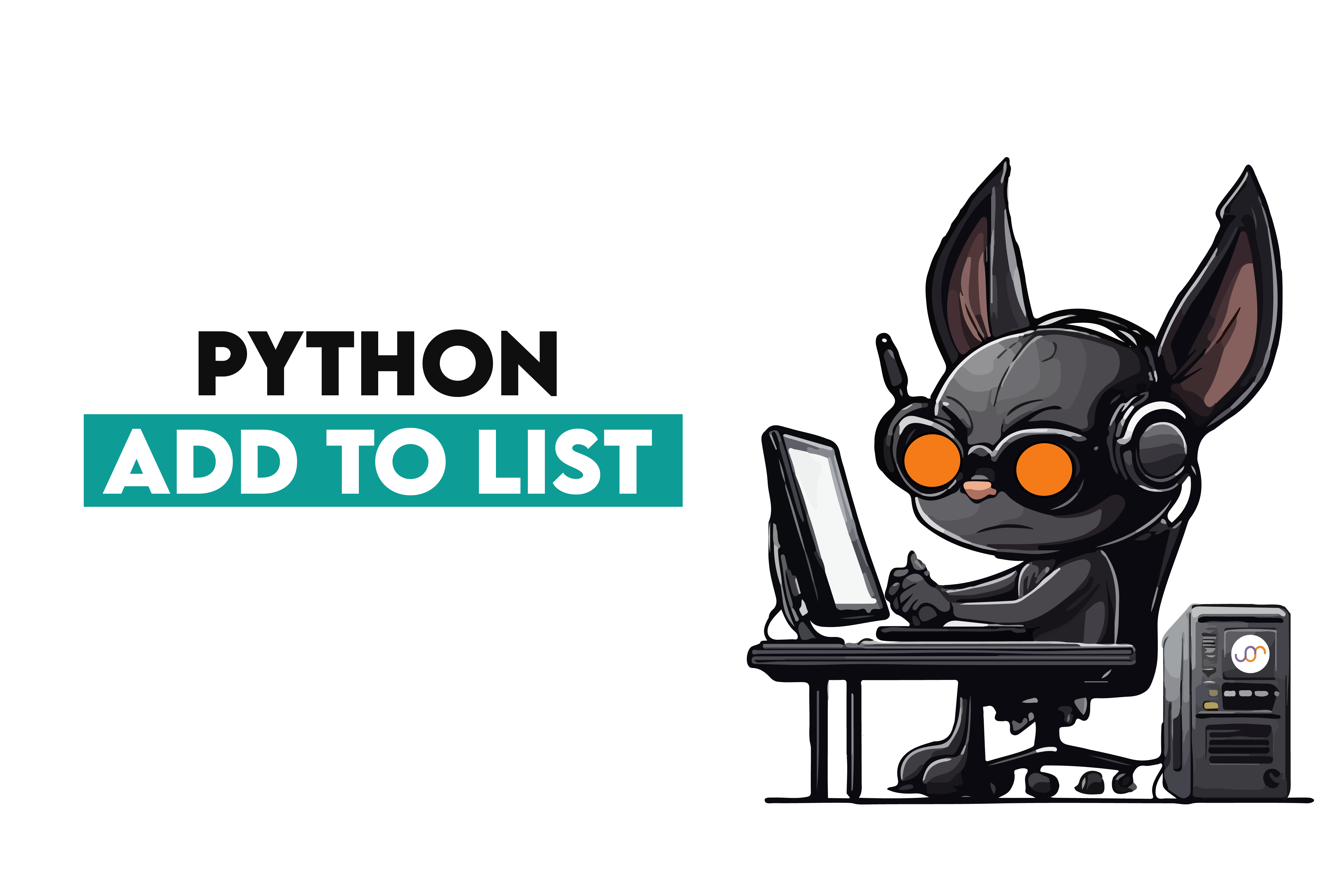
Categories:
- Written by:
Nathan Rosidi
Explore the versatile techniques of adding and removing elements in Python lists to enhance data manipulation and streamline your coding workflow.
Ever wondered how to handle better lists in Python? Lists manipulation is an integral part of any Python programmer.
In this guide, we will explore how to manipulate data by adding and removing elements from lists.
What is list manipulation in Python programming?
List manipulation uses the same specific operations as transforming a list's contents in Python. Lists are very dynamic, and you can easily add, remove, or change their data, making them a very useful tool for dealing with data in Python.
List manipulation includes:
- Elements to be added: Methods like append(), insert(), and extend() can be used to add elements to a list.
- Deleting Elements: You can delete elements from a list using methods such as remove(), pop(), and the del statement.
But let’s mention lists as another feature before starting.
Explanation of lists as ordered collections of items in Python
A list can have items with different data types, integers, floats, strings, and lists.Each element in a list is ordered, meaning it has a specific position. This is important for indexing and slicing operations.
Take, for example, the following list with a mix of data types.
my_list = [10, "apple", 3.14, True, [1, 2, 3]]
In this list:
- 10 is an integer
- "apple" is a string
- 3.14 is a float
- True is a boolean
- [1, 2, 3] is another list
Check this one about Python lists to discover it in depth.
Adding Elements to a List in Python
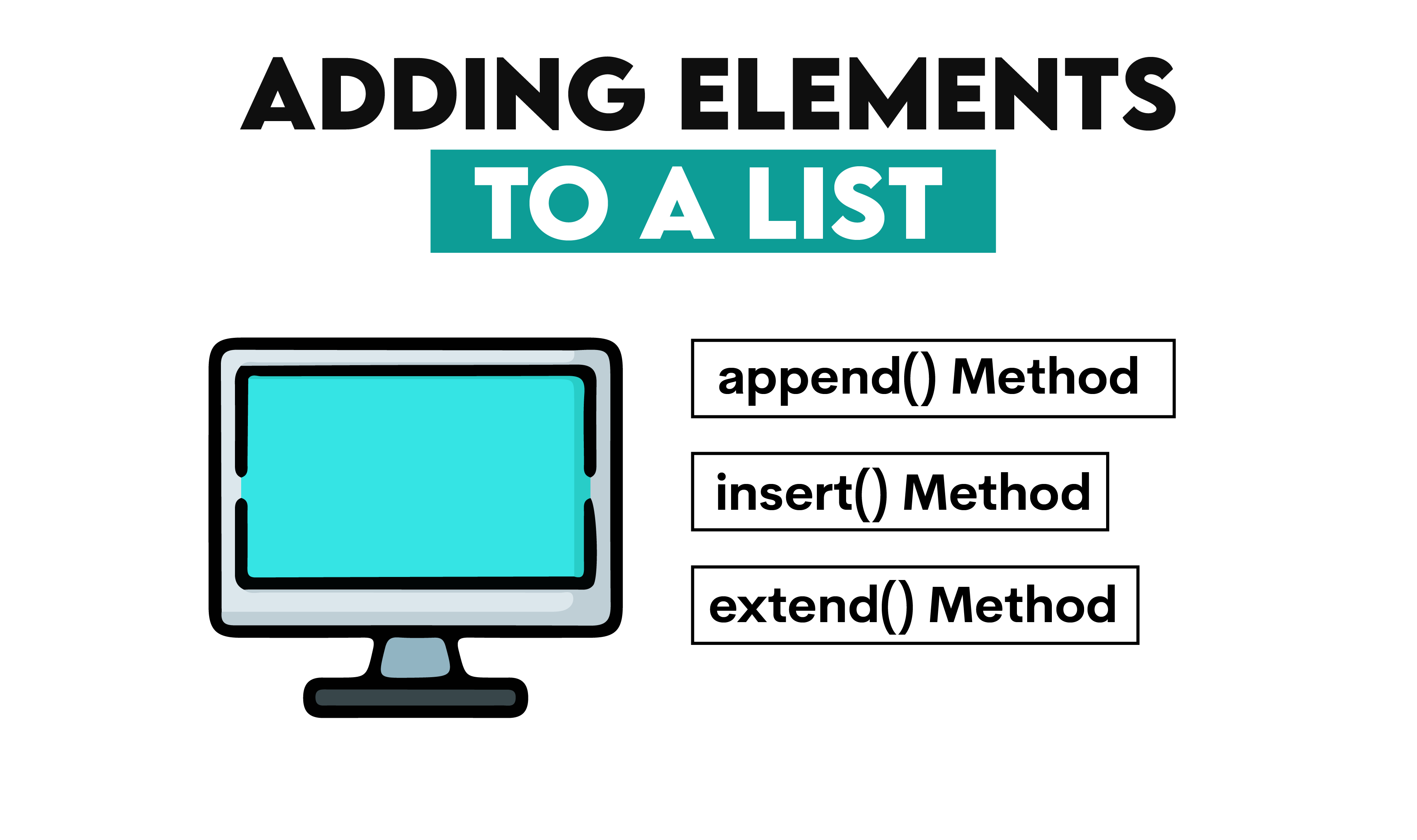
To add elements to a list, we can use append(), insert(), and extend() methods.
append() Method: Adding List Elements Individually in Python
The append() method in Python adds a single element to the end of a list. This method is especially useful when you need to dynamically build a list by adding new elements one at a time.
Consider the following question to understand this concept better.
Last Updated: April 2020
Calculate the running total (i.e., cumulative sum) energy consumption of the Meta/Facebook data centers in all 3 continents by the date. Output the date, running total energy consumption, and running total percentage rounded to the nearest whole number.
In this question, we are asked to find the cumulative sum of energy consumption data points and store these values in a list using Python's append() method.
Here is the link to this question: https://platform.stratascratch.com/coding/10084-cum-sum-energy-consumption
Now, let’s see the steps to solve this question.
Steps to Solve:
- Combine DataFrames: Merge DataFrames with energy consumption data using the append () method.
- Group by Date: In this final section, group the merged DataFrame by date to get an aggregate consumption based on Date.
- Cumulative Sum: Using the cumsum() method to calculate the sum of total consumption
- Calculate The consumption percentage of the total.
- Pre-process data: Drop the columns not used in this case and format the date column.
Let’s see the code.
import pandas as pd
import numpy as np
import datetime
# Assume fb_eu_energy, fb_na_energy, and fb_asia_energy are predefined DataFrames
merged_df = fb_eu_energy.append(fb_na_energy).append(fb_asia_energy)
# Step 2: Group by date and sum consumption
df = merged_df.groupby('date')['consumption'].sum().reset_index()
# Step 3: Calculate cumulative total consumption
df['cumulative_total_consumption'] = df['consumption'].cumsum()
# Step 4: Calculate percentage of total consumption
df['percentage_of_total_consumption'] = round((df['cumulative_total_consumption'] / df['consumption'].sum()) * 100)
# Step 5: Clean up data
df.drop("consumption", axis=1, inplace=True)
df['date'] = pd.to_datetime(df['date'], format='%Y-%m-%d').dt.strftime('%Y-%m-%d')
result = df
The resulting DataFrame df will contain the cumulative sums of the energy data points and the percentage of total consumption for each date:
This demonstrates how the append() method dynamically merges data from different sources to build a cumulative summary.
insert() Method: Adding List Elements at Specific Positions in Python
This is helpful when adding elements to an ordered list at specific positions. So, I want to delve into that concept with the following question:
Identify the most engaged guests by ranking them according to their overall messaging activity. The most active guest, meaning the one who has exchanged the most messages with hosts, should have the highest rank. If two or more guests have the same number of messages, they should have the same rank. Importantly, the ranking shouldn't skip any numbers, even if many guests share the same rank. Present your results in a clear format, showing the rank, guest identifier, and total number of messages for each guest, ordered from the most to least active.
Airbnb asked this question, and they want us to write the code to insert guest activity data at a specific position in a ranking list with the insert() in Python.
Follow this link for the question: https://platform.stratascratch.com/coding/10159-ranking-most-active-guests
Steps to Solve:
- Group By Guest ID and Sum Messages: Count all messages a guest sends.
- Value Counts: Count the messages per guest ID, then sort and reset their index.
- Rank Users: Give each user a placement carrying the messages they have sent.
- Insert Ranking Column: To add a ranking column at the start of DataFrame, use the insert() method.
Let’s see the code.
import pandas as pd
import numpy as np
df = airbnb_contacts.groupby('id_guest')['n_messages'].sum().reset_index().sort_values(['n_messages', 'id_guest'], ascending=[False, True])
df['ranking'] = df['n_messages'].rank(method='dense', ascending=False).astype(int)
my_column = df.pop('ranking')
df.insert(0, my_column.name, my_column)
result = df
print(result)
The resulting DataFrame df will show the guests ranked by their activity (number of messages), with the ranking inserted as the first column:
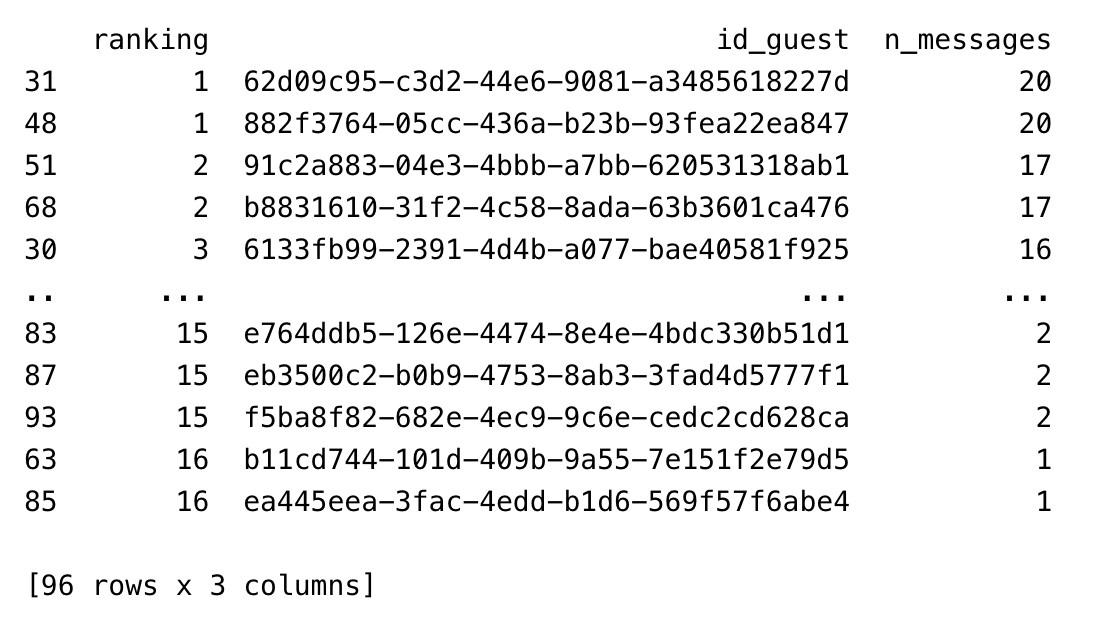
This showcases the insert() method's ability to precisely place elements (columns) within a DataFrame while maintaining a specific order.
You can check these python interview questions to know more about python and prepare an interview at the same time.
extend() Method: Adding List Elements from Another List in Python
The extend() method adds multiple elements to the end of a list. It comes in handy when you combine two lists or insert a collection of elements into an existing list.
Suppose you have the region-wise sales data for multiple products, and you have to merge these lists and analyze the consolidated list of the combined sales data.
Steps to Solve:
- Load Data: Two lists that contain data on sales region-wise.
- Append Both Lists together: Using the extend() method, add elements from the second List into the first List.
- Process the Combined Data: This is the stage where you may analyze the data further in-depth using other methods, such as calculating total sales or the top-selling product.
Here is the code.
import pandas as pd
import numpy as np
region1_sales = [("product1", 250), ("product2", 300), ("product3", 400)]
region2_sales = [("product4", 150), ("product5", 500), ("product6", 600)]
region1_sales.extend(region2_sales)
print(region1_sales)
The output list region1_sales will now contain all elements from both lists.
This highlights how the extend() method efficiently merges two lists into one. Check this one to learn more about Python list methods.
Removing Elements from a List in Python

To do that, we’ll use three different methods: remove(), pop(), and del. Let’s start with remove!
remove() Method: Removing List Elements by Value in Python
In Python, the remove() method removes the first occurrence of the specified value from the list. This method is helpful when you want to delete a selected element by its value.
Now, consider a scenario where you have a list of product names and must delete a discontinued product.
Steps to Solve
- Create the List: A list containing product names.
- Find The Element: The name of the product to be removed.
- Remove The Element: Remove the product from a list at the specified location using remove().
product_ids = ["product1", "product2", "product3", "product4", "product5"]
discontinued_product = "product3"
product_ids.remove(discontinued_product)
print(product_ids)
The output list will show the list after the specified product has been removed:
pop() method: Removing List Elements from the End in Python
Pop() removes and returns the final element without regard to the index.
Let’s say you have a dataset consisting of customer orders. You wish to remove the last item on this list of client orders.
Steps to Solve:
- Initialize the list: This will start with the list of customers or orders.
- Describe where it is: if not the last, tell in which position this element is.
- Remove and Get the Element: Remove and get the element from the list, which can be done using the pop() method.
customer_orders = ["order1", "order2", "order3", "order4", "order5"]
last_order = customer_orders.pop()
print("Updated Orders List:", customer_orders)
print("Processed Order:", last_order)
The output list customer_orders will show the list after the last order has been processed and removed, and the removed order ID will be displayed:
This demonstrates how the pop() method retrieves and removes the last element from the list.
del statement: Removing List Elements by Index in Python
Python uses a del statement—which removes objects from a list based on their locations or whole variables.
Assume you have a product list and wish to exclude specific items by indicating their indices.
Steps to Solve:
- Creating List: An initialization of the list of products needs to be made.
- Positions: Find the products to be deleted.
- Deletion: Use the del() method.
Here is the code.
products = ["product1", "product2", "product3", "product4", "product5"]
indices_to_remove = [1, 3]
del products[indices_to_remove[0]]
del products[indices_to_remove[1] - 1]
print(products)
The output list products will show the list after the specified products have been removed:
This demonstrates how the del statement deletes elements from a list by their index.
Best Practices tips to prevent bugs and enhance code readability
When working with Python, careful list handling can help to save a great deal of future trouble. Let us consider some instances, then.
- Name Clearly: For a variable storing data, say sales_data instead of sd using a meaningful name.
- Use List Comprehensions: List comprehensions make your code neat and simple; hence, you should always choose them if you are building a list.
- Notes Your Code: Comments clarifying intricate processes' goals will help you grasp your code.
- Formatting Consistently: Keep indentation and formatting consistent in your code to make it easier to read.
- Avoid Modifying Lists in Place: Modify lists in place only when required, and be cautious, as this can create undesired side effects. Let's start a new list instead.
Conclusion
To summarize, add and remove the elements to the lists in Python in the best way, use append(), insert(), extend(), remove(), pop(), del insertions, and deletions in Python.
Further exploration and practice with these methods will enhance your Python programming skills. StrataScratch provides a wealth of resources and real-world problems to practice these.
Share